void int0() interrupt 0 { uchar i; for (i = 0; i < 10; i++) { P0 = tab2[i]; delay(); } }
时间: 2024-05-29 07:11:43 浏览: 11
This code defines an interrupt service routine for external interrupt 0 (INT0). When the interrupt is triggered, the code will execute a loop that iterates 10 times. During each iteration, the value of the variable i will be used as an index to access an element of the array tab2, and that value will be written to P0. After each write to P0, there will be a delay before the next iteration of the loop.
However, there is a mistake in the code - the incrementation of i is missing from the for loop's expression. As it stands, the loop will never terminate and will cause the program to hang. The correct expression should be "i++" to increment i by 1 after each iteration.
相关问题
void int0() interrupt 0 { uchar i; for (i = 0; i < 10; i++) { P0 = tab2[i]; delay(); } }解释这段代码
这段代码是中断0的中断服务函数,当中断0触发时,即外部中断0引脚有信号改变时,该函数会被自动调用。其中,该函数会循环输出数组tab2中的元素到P0口,并通过delay()函数产生一定的延时。
具体而言,该函数会定义一个uchar类型的变量i,初始值为0,然后通过for循环,循环10次,每次循环将i自增1,即i++。在循环中,每次将tab2数组的第i个元素输出到P0口,并通过delay()函数产生一定的延时。因此,该函数的作用是在外部中断0触发时,循环输出tab2数组的元素到P0口。
#include<reg52.h> #include<intrins.h> #define uchar unsigned char uchar code tab[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8, 0x80,0x90,0x88,0x83,0xc6,0xa1,0x86,0x8e}; int i=5000; char dir=0; char count=1; char count1=0; void main() { TMOD=0x20; TL1=56; TH1=56; P1=0xfe; EA=1; ET1=1; TR1=1; while(1) { P0=tab[count1]; }; } void timer1() interrupt 3 { i--; if(i==2500) { P1=0xff; } if(i==0&&dir==0) { P1=_crol_(0xfe,count); count++; i=5000; if(count==8) { dir=1; count=1; count1++; } } if(i==0&&dir==1) { P1=_cror_(0x7f,count); count++; i=5000; if(count==8) { dir=0; count=1; count1++; } } if(count1==16) { count1=0; } }解释这个代码
这段代码是用来控制一个8位LED流水灯的程序。下面是代码的解释:
```C
#include<reg52.h>
#include<intrins.h>
#define uchar unsigned char
uchar code tab[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,
0x80,0x90,0x88,0x83,0xc6,0xa1,0x86,0x8e};
```
这里定义了一个字符型常量数组`tab`,存储了0~F在共阴数码管上的显示值。
```C
int i=5000;
char dir=0;
char count=1;
char count1=0;
```
定义了一个定时器计数器`i`,一个方向标志位`dir`,一个计数器`count`,用于计算改变方向的次数,一个计数器`count1`,用于切换数码管上的数字。
```C
void main() {
TMOD=0x20;
TL1=56;
TH1=56;
P1=0xfe;
EA=1;
ET1=1;
TR1=1;
while(1) {
P0=tab[count1];
};
}
```
在主函数中,我们设置了定时器1为模式2,定时器初值为56,计数器P1初始值为0xfe。开启定时器1中断,并开启总中断。
```C
void timer1() interrupt 3 {
i--;
if(i==2500) {
P1=0xff;
}
if(i==0&&dir==0) {
P1=_crol_(0xfe,count);
count++;
i=5000;
if(count==8) {
dir=1;
count=1;
count1++;
}
}
if(i==0&&dir==1) {
P1=_cror_(0x7f,count);
count++;
i=5000;
if(count==8) {
dir=0;
count=1;
count1++;
}
}
if(count1==16) {
count1=0;
}
}
```
这里定义了定时器1中断服务函数。在函数中,我们先将定时器计数器`i`减1,如果计数器`i`的值为2500,将P1的值设为0xff,即LED流水灯全灭。如果计数器`i`的值为0并且方向标志位`dir`为0,将P1的值左移一位,将计数器`count`加1,将计数器`i`的值设为5000,如果计数器`count`的值等于8,则将方向标志位`dir`设为1,将计数器`count`的值设为1,将计数器`count1`加1。如果计数器`i`的值为0并且方向标志位`dir`为1,将P1的值右移一位,将计数器`count`加1,将计数器`i`的值设为5000,如果计数器`count`的值等于8,则将方向标志位`dir`设为0,将计数器`count`的值设为1,将计数器`count1`加1。如果计数器`count1`的值等于16,则将计数器`count1`的值设为0。
这段代码实现了一个8位的LED流水灯,每次改变方向都会让数码管上的数字加1,当数码管上的数字等于F时,再次从0开始。
相关推荐
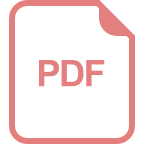
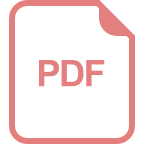










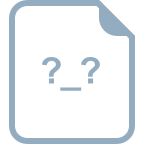