C#定义圆类Circle,包含成员变量:半径radius及其对应的属性。公共方法有。面积方法Area(),打印输出方法Printinfo。从圆类中派生出球体类Sphere、圆柱类Cylinder和圆锥类Cube。在主函数中分别实例化以上类的实例,调用相应的方法,计算并输出面积和体积。
时间: 2023-11-22 12:54:44 浏览: 61
以下是C#定义圆类Circle,包含成员变量:半径radius及其对应的属性。公共方法有。面积方法Area(),打印输出方法Printinfo。从圆类中派生出球体类Sphere、圆柱类Cylinder和圆锥类Cube。在主函数中分别实例化以上类的实例,调用相应的方法,计算并输出面积和体积的示例代码:
```csharp
using System;
// 定义圆类
public class Circle
{
private double radius; // 半径
// 半径属性
public double Radius
{
get { return radius; }
set { radius = value; }
}
// 面积方法
public double Area()
{
return Math.PI * radius * radius;
}
// 打印输出方法
public void Printinfo()
{
Console.WriteLine("圆的半径为:" + radius);
Console.WriteLine("圆的面积为:" + Area());
}
}
// 派生球体类
public class Sphere : Circle
{
// 体积方法
public double Volume()
{
return 4.0 / 3.0 * Math.PI * Math.Pow(Radius, 3);
}
// 打印输出方法
public void Printinfo()
{
Console.WriteLine("球的半径为:" + Radius);
Console.WriteLine("球的体积为:" + Volume());
}
}
// 派生圆柱类
public class Cylinder : Circle
{
private double height; // 高
// 高属性
public double Height
{
get { return height; }
set { height = value; }
}
// 体积方法
public double Volume()
{
return Math.PI * Radius * Radius * height;
}
// 打印输出方法
public void Printinfo()
{
Console.WriteLine("圆柱的半径为:" + Radius);
Console.WriteLine("圆柱的高为:" + height);
Console.WriteLine("圆柱的体积为:" + Volume());
}
}
// 派生圆锥类
public class Cone : Circle
{
private double height; // 高
// 高属性
public double Height
{
get { return height; }
set { height = value; }
}
// 体积方法
public double Volume()
{
return 1.0 / 3.0 * Math.PI * Radius * Radius * height;
}
// 打印输出方法
public void Printinfo()
{
Console.WriteLine("圆锥的半径为:" + Radius);
Console.WriteLine("圆锥的高为:" + height);
Console.WriteLine("圆锥的体积为:" + Volume());
}
}
class Program
{
static void Main(string[] args)
{
// 实例化圆类
Circle circle = new Circle();
circle.Radius = 2.0;
circle.Printinfo();
// 实例化球体类
Sphere sphere = new Sphere();
sphere.Radius = 3.0;
sphere.Printinfo();
// 实例化圆柱类
Cylinder cylinder = new Cylinder();
cylinder.Radius = 2.0;
cylinder.Height = 5.0;
cylinder.Printinfo();
// 实例化圆锥类
Cone cone = new Cone();
cone.Radius = 3.0;
cone.Height = 4.0;
cone.Printinfo();
Console.ReadKey();
}
}
```
阅读全文
相关推荐
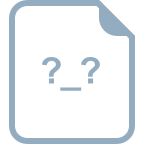
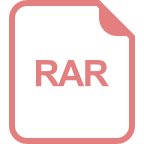
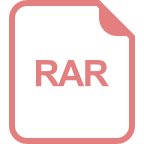
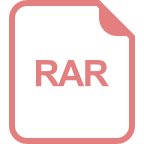
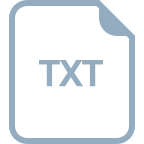
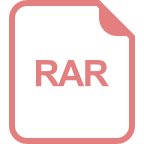
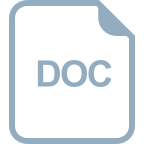
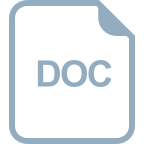
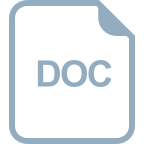
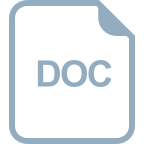
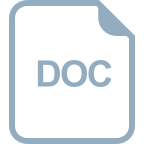



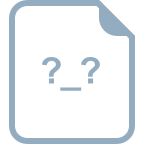
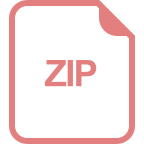
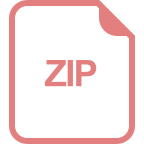