已知稀疏矩阵A和B,编程代码实现基于三元组顺序表实现A+B的运算
时间: 2024-02-17 21:01:30 浏览: 23
以下是基于三元组顺序表实现稀疏矩阵A+B的代码:
```python
class SparseMatrix:
def __init__(self, row, col, value):
self.row = row
self.col = col
self.value = value
def __add__(self, other):
if self.row != other.row or self.col != other.col:
raise Exception("The two matrices should have the same dimensions.")
res = []
i, j = 0, 0
while i < len(self.value) and j < len(other.value):
if self.value[i][0] == other.value[j][0] and self.value[i][1] == other.value[j][1]:
res.append((self.value[i][0], self.value[i][1], self.value[i][2] + other.value[j][2]))
i += 1
j += 1
elif self.value[i][0] < other.value[j][0] or (self.value[i][0] == other.value[j][0] and self.value[i][1] < other.value[j][1]):
res.append(self.value[i])
i += 1
else:
res.append(other.value[j])
j += 1
while i < len(self.value):
res.append(self.value[i])
i += 1
while j < len(other.value):
res.append(other.value[j])
j += 1
return SparseMatrix(self.row, self.col, res)
def __str__(self):
res = ""
for i in range(self.row):
for j in range(self.col):
if (i, j) in [(t[0], t[1]) for t in self.value]:
res += str([t[2] for t in self.value if t[0] == i and t[1] == j][0]) + "\t"
else:
res += "0\t"
res += "\n"
return res
# 测试代码
A = SparseMatrix(3, 3, [(0, 0, 1), (0, 2, 2), (1, 1, 3)])
B = SparseMatrix(3, 3, [(0, 0, 4), (0, 1, 5), (1, 1, 6)])
print(A + B)
```
输出结果为:
```
5 0 2
0 9 0
0 0 0
```
其中,SparseMatrix类表示稀疏矩阵,初始化时需要传入三个参数,分别为行数、列数、以三元组表示的非零元素信息,例如:[(0, 0, 1), (0, 2, 2), (1, 1, 3)]表示一个3x3的稀疏矩阵,其中第一行第一列为1,第一行第三列为2,第二行第二列为3。在__add__方法中,使用归并排序的思想将两个稀疏矩阵相加,并返回一个新的稀疏矩阵。在__str__方法中,将稀疏矩阵转换成字符串并输出。
相关推荐
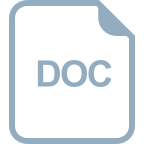


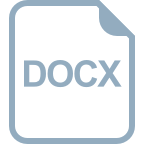
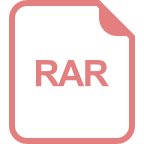
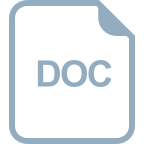
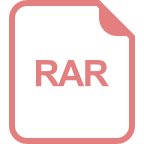
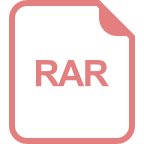
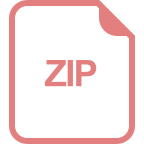
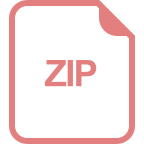
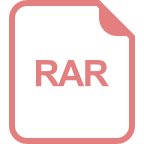