请使用python实现:计算并绘制期权的二维和三维波动率微笑曲线
时间: 2023-08-11 12:03:41 浏览: 228
好的,我可以为您提供一个基本的Python代码框架,用于计算和绘制期权的二维和三维波动率微笑曲线。请注意,这个代码框架需要一些期权定价模型和数据源的支持,这些需要根据具体需求自行添加。
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 定义期权定价模型和数据源
# 定义波动率计算函数
def calc_volatility(option, underlying_price, risk_free_rate, time_to_maturity):
# 根据期权定价模型计算期权价格
option_price = ...
# 根据期权价格反推波动率
volatility = ...
return volatility
# 计算二维波动率微笑曲线
def calc_smile_surface():
# 定义计算参数范围和步长
underlying_prices = np.arange(...)
time_to_maturities = np.arange(...)
# 创建空的二维数组,用于存储波动率数据
volatilities = np.zeros((len(underlying_prices), len(time_to_maturities)))
# 遍历参数范围,计算波动率
for i in range(len(underlying_prices)):
for j in range(len(time_to_maturities)):
volatilities[i, j] = calc_volatility(option, underlying_prices[i], risk_free_rate, time_to_maturities[j])
# 绘制二维波动率微笑曲线
fig = plt.figure()
ax = fig.add_subplot(111)
ax.plot(...)
ax.set_xlabel('Underlying Price')
ax.set_ylabel('Volatility')
ax.set_title('Volatility Smile')
plt.show()
# 计算三维波动率微笑曲面
def calc_smile_curve():
# 定义计算参数范围和步长
underlying_prices = np.arange(...)
time_to_maturities = np.arange(...)
# 创建空的三维数组,用于存储波动率数据
volatilities = np.zeros((len(underlying_prices), len(time_to_maturities)))
# 遍历参数范围,计算波动率
for i in range(len(underlying_prices)):
for j in range(len(time_to_maturities)):
volatilities[i, j] = calc_volatility(option, underlying_prices[i], risk_free_rate, time_to_maturities[j])
# 绘制三维波动率微笑曲面
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(...)
ax.set_xlabel('Underlying Price')
ax.set_ylabel('Time to Maturity')
ax.set_zlabel('Volatility')
ax.set_title('Volatility Smile')
plt.show()
# 调用计算函数,绘制波动率微笑曲线
calc_smile_surface()
calc_smile_curve()
```
请注意,这个代码框架只是一个示例,需要在实际使用中根据具体需求进行修改和完善。同时,需要注意期权定价模型和数据源的选择和准确性,以确保计算结果的可靠性。
阅读全文
相关推荐
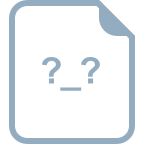
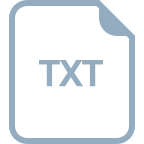
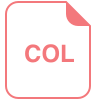
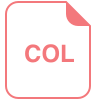
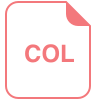
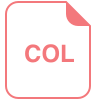
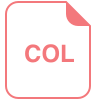
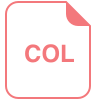
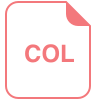
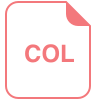
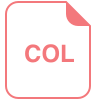
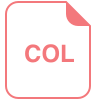
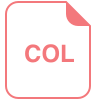
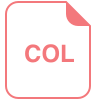
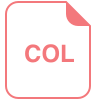
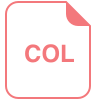