Vue 实现输入框短信验证码功能
时间: 2023-10-06 20:14:09 浏览: 126
实现输入框短信验证码功能,可以使用 Vue.js 的模板语法和组件化思想,具体实现步骤如下:
1. 创建一个包含输入框和发送验证码按钮的组件。
```html
<template>
<div>
<input type="text" v-model="code" />
<button @click="sendCode" :disabled="disabled">{{ buttonText }}</button>
</div>
</template>
<script>
export default {
data() {
return {
code: '',
disabled: false,
buttonText: '发送验证码',
countdown: 60
}
},
methods: {
sendCode() {
// 发送短信验证码
// ...
// 设置倒计时
this.disabled = true;
this.buttonText = `${this.countdown}s 后重新发送`;
const timer = setInterval(() => {
this.countdown--;
this.buttonText = `${this.countdown}s 后重新发送`;
if (this.countdown === 0) {
clearInterval(timer);
this.disabled = false;
this.buttonText = '发送验证码';
this.countdown = 60;
}
}, 1000);
}
}
}
</script>
```
2. 在父组件中引入该组件,并监听子组件的输入事件。
```html
<template>
<div>
<sms-code @input="handleInput" />
<button @click="submit">提交</button>
</div>
</template>
<script>
import SmsCode from './SmsCode.vue';
export default {
components: {
SmsCode
},
data() {
return {
phone: '',
code: ''
}
},
methods: {
handleInput(value) {
this.code = value;
},
submit() {
// 提交表单
// ...
}
}
}
</script>
```
在子组件中,使用 `v-model` 来实现与父组件的双向数据绑定。当输入框的值发生变化时,通过 `$emit` 方法将新的值传递给父组件。
```html
<template>
<div>
<input type="text" v-model="code" @input="$emit('input', code)" />
<button @click="sendCode" :disabled="disabled">{{ buttonText }}</button>
</div>
</template>
<script>
export default {
data() {
return {
code: '',
disabled: false,
buttonText: '发送验证码',
countdown: 60
}
},
methods: {
sendCode() {
// 发送短信验证码
// ...
// 设置倒计时
this.disabled = true;
this.buttonText = `${this.countdown}s 后重新发送`;
const timer = setInterval(() => {
this.countdown--;
this.buttonText = `${this.countdown}s 后重新发送`;
if (this.countdown === 0) {
clearInterval(timer);
this.disabled = false;
this.buttonText = '发送验证码';
this.countdown = 60;
}
}, 1000);
}
}
}
</script>
```
这样就可以实现一个简单的输入框短信验证码功能了。
阅读全文
相关推荐
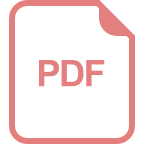
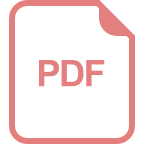
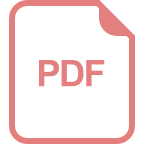
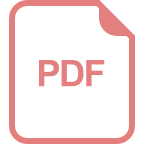
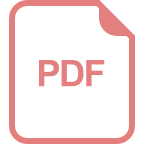
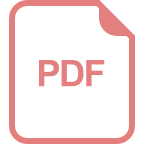
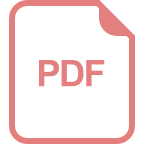
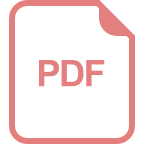
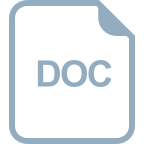
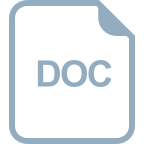
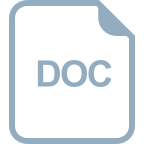



