用c语言编写一个停车场的小程序并把运行结果写出来
时间: 2024-12-25 22:28:09 浏览: 22
在C语言中创建一个简单的停车场小程序可以涉及一些基本的数据结构和用户交互。这里是一个简化的例子,它使用数组模拟有限的停车位,用户可以查看剩余车位,停车和取车:
```c
#include <stdio.h>
#define MAX_PARKINGSLOTS 5 // 最大停车位数
int parkinglots[MAX_PARKINGSLOTS]; // 停车位数组,初始化为0表示空
void print_slots() {
int i;
printf("Current parking slots:\n");
for (i = 0; i < MAX_PARKINGSLOTS; i++) {
if (parkinglots[i] == 1) {
printf("%d. Parked\n", i + 1);
} else {
printf("%d. Empty\n", i + 1);
}
}
}
void park(int slot) {
if (slot >= 0 && slot < MAX_PARKINGSLOTS && parkinglots[slot] == 0) {
parkinglots[slot] = 1;
printf("Car parked at slot %d.\n", slot + 1);
} else {
printf("Slot %d is either full or out of range.\n", slot + 1);
}
}
void leave(int slot) {
if (slot >= 0 && slot < MAX_PARKINGSLOTS && parkinglots[slot] == 1) {
parkinglots[slot] = 0;
printf("Car taken from slot %d.\n", slot + 1);
} else {
printf("No car in slot %d.\n", slot + 1);
}
}
int main() {
while (1) {
int choice, slot;
printf("\nChoose an option:\n");
printf("1. View current slots\n");
printf("2. Park a car\n");
printf("3. Leave a car\n");
printf("4. Exit program\n");
scanf("%d", &choice);
switch (choice) {
case 1:
print_slots();
break;
case 2:
printf("Enter the parking slot number: ");
scanf("%d", &slot);
park(slot - 1); // 减1是因为索引从0开始
break;
case 3:
printf("Enter the parking slot number: ");
scanf("%d", &slot);
leave(slot - 1);
break;
case 4:
exit(0); // 结束程序
break;
default:
printf("Invalid choice.\n");
break;
}
}
return 0;
}
```
这个程序通过`main()`函数接收用户输入,然后调用相应的函数(`print_slots()`、`park()` 和 `leave()`)。当用户选择退出时,程序结束。
注意,这只是一个非常基础的示例,实际的停车场程序可能会更复杂,包括错误检查、用户界面优化和持久化数据存储等功能。
阅读全文
相关推荐






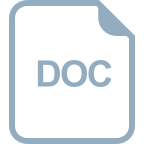
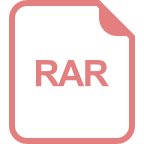










