用c语言给我编写一个停车场的小程序代码
时间: 2024-12-24 18:28:15 浏览: 20
为了创建一个简单的停车场管理小程序,我们将使用C语言的基本结构来模拟车辆的停车和取车过程。这里是一个基本的控制台版示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX ParkingLots // 假设最大停车位数
typedef struct {
int id; // 车辆ID
int status; // 状态(0表示空闲,1表示占用)
} Car;
Car parking_lots[MAX]; // 停车位数组
int park(int vehicle_id) {
if (find_empty_slot()) { // 查找空闲车位
parking_lots[slot_index].id = vehicle_id;
parking_lots[slot_index].status = 1; // 设置为占用状态
printf("Vehicle %d parked successfully at slot %d.\n", vehicle_id, slot_index);
return 1; // 成功返回
} else {
printf("No available slots. Can't park the vehicle.\n");
return 0; // 无空位返回
}
}
int take_car(int vehicle_id) {
for (int i = 0; i < MAX; i++) {
if (parking_lots[i].id == vehicle_id && parking_lots[i].status == 1) { // 找到对应车辆
parking_lots[i].status = 0; // 取消占用
printf("Vehicle %d taken from slot %d.\n", vehicle_id, i);
return 1; // 成功返回
}
}
printf("Cannot find vehicle with ID %d.\n", vehicle_id);
return 0; // 未找到车辆返回
}
// 模拟查找空闲车位函数,这里简化只考虑数组遍历
int find_empty_slot() {
for (int i = 0; i < MAX; i++) {
if (parking_lots[i].status == 0)
return i; // 返回第一个空闲位置
}
return -1; // 如果全满则返回-1
}
int main() {
int choice, option;
while (1) {
printf("\n1. Park a car\n2. Take a car\n3. Exit\nEnter your choice: ");
scanf("%d", &choice);
switch(choice) {
case 1:
printf("Enter vehicle ID: ");
scanf("%d", &option);
if (park(option))
break;
// 没有跳出循环继续下一轮操作
case 2:
printf("Enter vehicle ID to take: ");
scanf("%d", &option);
if (take_car(option))
break;
// 同理,如果成功取车则退出当前选择
case 3:
exit(0); // 退出程序
break;
default:
printf("Invalid choice, try again.\n");
}
}
return 0;
}
```
这个程序非常基础,仅用于演示停车场的基本功能。实际应用中,可能会需要数据库支持、错误处理等更复杂的功能。
阅读全文
相关推荐


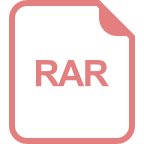




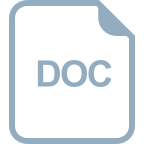









