from concurrent.futures import ThreadPoolExecutor from selenium import webdriver from selenium.webdriver.chrome.options import Options # 打开URL文件 with open('url.txt', 'r') as file: urls = file.read().splitlines() # 定义特定的域名 specific_domains = ['4qo4li.com:9516/register?i_code='] # 创建ChromeOptions对象 chrome_options = Options() chrome_options.add_argument("--incognito") # 启用无痕模式 def process_url(url): # 创建浏览器实例 driver = webdriver.Chrome(options=chrome_options) # 使用Chrome浏览器,需要下载对应的驱动并设置到环境变量中 # 构建完整的URL full_url = 'http://' + url + '/a/index.php/54545' # 打开浏览器并访问URL driver.get(full_url) # 等待页面跳转完成 driver.implicitly_wait(10) # 设置隐式等待时间,单位为秒 # 获取当前页面的URL current_url = driver.current_url # 判断当前页面的URL是否包含特定域名 if any(domain in current_url for domain in specific_domains): # 写入1.txt文本文件 with open('1.txt', 'a') as file: file.write(url + '\n') else: # 写入2.txt文本文件 with open('2.txt', 'a') as file: file.write(url + '\n') # 关闭浏览器 driver.quit() # 创建线程池 with ThreadPoolExecutor(max_workers=10) as executor: # 提交任务给线程池 futures = [executor.submit(process_url, url) for url in urls] # 等待所有任务完成 for future in futures: future.result()
时间: 2024-04-17 15:27:38 浏览: 137
这段代码使用了Python的concurrent.futures库来创建了一个线程池,并使用Selenium库来进行网页操作。代码首先从url.txt文件中读取URL列表,然后定义了一个特定的域名列表。接下来,创建了一个ChromeOptions对象,添加了启用无痕模式的参数。然后,定义了一个process_url函数,该函数用于处理每个URL。在函数内部,首先创建了一个Chrome浏览器实例,然后构建完整的URL并打开浏览器访问该URL。然后等待页面跳转完成,获取当前页面的URL,并判断该URL是否包含特定域名。根据判断结果,将URL写入不同的文件中。最后,关闭浏览器。接下来,使用ThreadPoolExecutor创建了一个最大线程数为10的线程池,并将任务提交给线程池进行处理。最后,等待所有任务完成。
请问有什么问题我可以帮助您解答吗?
相关问题
再次处理failed_urls.txt中失败的URL 判断是否仍然失败,写入2.txt文件
要再次处理`failed_urls.txt`中的失败URL,并判断是否仍然失败,将仍然失败的URL写入`2.txt`文件中,可以进行如下修改:
```python
from concurrent.futures import ThreadPoolExecutor, wait
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
# 打开URL文件
with open('url.txt', 'r') as file:
urls = file.read().splitlines()
# 定义特定的域名
specific_domains = ['4qo4li.com:9516/register?i_code=']
# 创建ChromeOptions对象
chrome_options = Options()
chrome_options.add_argument("--incognito") # 启用无痕模式
def process_url(url):
# 创建浏览器实例
driver = webdriver.Chrome(options=chrome_options) # 使用Chrome浏览器,需要下载对应的驱动并设置到环境变量中
# 构建完整的URL
full_url = 'http://' + url + '/a/index.php/54545'
# 打开浏览器并访问URL
driver.get(full_url)
# 等待页面跳转完成
driver.implicitly_wait(10) # 设置隐式等待时间,单位为秒
# 获取当前页面的URL
current_url = driver.current_url
# 判断当前页面的URL是否包含特定域名
if any(domain in current_url for domain in specific_domains):
# 写入1.txt文本文件
with open('1.txt', 'a') as file:
file.write(url + '\n')
else:
# 写入2.txt文本文件
with open('2.txt', 'a') as file:
file.write(url + '\n')
# 关闭浏览器
driver.quit()
# 创建线程池
with ThreadPoolExecutor(max_workers=10) as executor:
# 提交任务给线程池
futures = [executor.submit(process_url, url) for url in urls]
# 等待所有任务完成
wait(futures)
# 打开failed_urls.txt文件
with open('failed_urls.txt', 'r') as file:
failed_urls = file.read().splitlines()
# 创建新的线程池用于处理失败的URL
with ThreadPoolExecutor(max_workers=10) as executor:
# 提交任务给线程池
futures = [executor.submit(process_url, url) for url in failed_urls]
# 等待所有任务完成
wait(futures)
# 将仍然失败的URL写入2.txt文件中
for future, url in zip(futures, failed_urls):
if future.exception() is not None:
with open('2.txt', 'a') as file:
file.write(url + '\n')
```
在代码中,首先使用线程池处理原始的URL列表。然后,打开`failed_urls.txt`文件,读取其中的失败URL,并使用新的线程池处理这些URL。在处理完所有失败URL后,通过检查每个任务的异常状态来判断是否仍然失败,将仍然失败的URL写入`2.txt`文件中。
希望这个修改能满足您的需求。如果还有其他问题,请随时提问。
在遍历完所有URL后,再次打开浏览器窗口来处理失败的URL,如果还是失败将其写入2.txt文件中
要在遍历完所有URL后再次打开浏览器窗口来处理失败的URL,并将仍然失败的URL写入2.txt文件中,可以使用一个额外的列表来存储失败的URL,然后在所有任务完成后,重新处理这些失败的URL。
以下是修改后的代码示例:
```python
from concurrent.futures import ThreadPoolExecutor
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
# 打开URL文件
with open('url.txt', 'r') as file:
urls = file.read().splitlines()
# 定义特定的域名
specific_domains = ['4qo4li.com:9516/register?i_code=']
# 创建ChromeOptions对象
chrome_options = Options()
chrome_options.add_argument("--incognito") # 启用无痕模式
def process_url(url):
# 创建浏览器实例
driver = webdriver.Chrome(options=chrome_options) # 使用Chrome浏览器,需要下载对应的驱动并设置到环境变量中
# 构建完整的URL
full_url = 'http://' + url + '/a/index.php/54545'
# 打开浏览器并访问URL
driver.get(full_url)
# 等待页面跳转完成
driver.implicitly_wait(10) # 设置隐式等待时间,单位为秒
# 获取当前页面的URL
current_url = driver.current_url
# 判断当前页面的URL是否包含特定域名
if any(domain in current_url for domain in specific_domains):
# 写入1.txt文本文件
with open('1.txt', 'a') as file:
file.write(url + '\n')
else:
# 添加到失败的URL列表
failed_urls.append(url)
# 关闭浏览器
driver.quit()
# 创建线程池
with ThreadPoolExecutor(max_workers=10) as executor:
# 提交任务给线程池
futures = [executor.submit(process_url, url) for url in urls]
# 等待所有任务完成
for future in futures:
future.result()
# 再次处理失败的URL
failed_urls = []
for url in failed_urls:
process_url(url)
# 判断是否仍然失败,写入2.txt文件
if url in failed_urls:
with open('2.txt', 'a') as file:
file.write(url + '\n')
```
在代码中,我添加了一个名为`failed_urls`的列表,用于存储处理失败的URL。在处理每个URL时,如果判断为失败,则将该URL添加到`failed_urls`列表中。在所有任务完成后,遍历`failed_urls`列表,再次调用`process_url`函数来重新处理这些失败的URL。然后,判断是否仍然失败,如果仍然失败,则将该URL写入2.txt文件中。
希望这个修改能满足您的需求。如果还有其他问题,请随时提问。
阅读全文
相关推荐
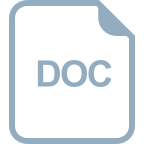
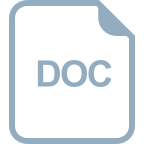
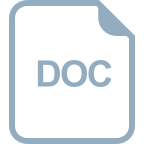
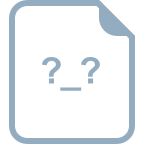
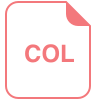
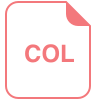
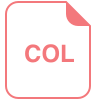
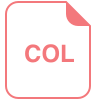
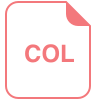
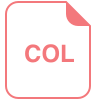
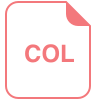
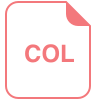




