混合模拟退火遗传算法python
时间: 2023-10-19 21:07:00 浏览: 111
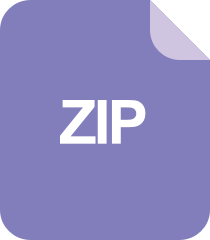
模拟退火算法与遗传算法_遗传模拟退火_遗传和模拟退火算法_seldomqwf_遗传退火算法_
混合模拟退火遗传算法是一种结合了模拟退火算法和遗传算法的优化算法。它利用遗传算法的优点进行全局搜索和局部搜索,同时结合模拟退火算法的随机性进行解空间的探索。
下面是混合模拟退火遗传算法的步骤:
1. 初始化种群:随机生成一组初始个体作为初始种群。
2. 计算适应度:对每一个个体计算适应度值。
3. 判断停止条件:判断是否满足停止条件,如果满足则输出最优解,否则继续执行以下步骤。
4. 选择操作:根据适应度值大小以一定方式执行选择操作,选择出一部分个体作为父代。
5. 交叉操作:对父代个体进行交叉操作,生成新的子代个体。
6. 变异操作:对子代个体进行变异操作,引入新的基因变化。
7. 更新种群:将新的子代个体加入种群中,并删除一部分旧的个体。
8. 返回步骤2。
这样通过不断的选择、交叉和变异操作,混合模拟退火遗传算法能够在解空间中进行全局搜索,并通过模拟退火的随机性进行局部搜索,从而找到较优解。
阅读全文
相关推荐
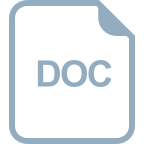
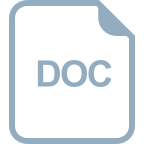
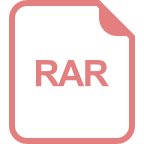
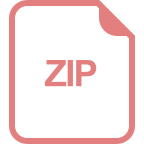
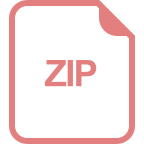
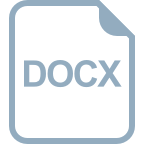
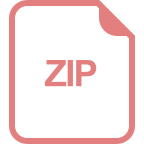
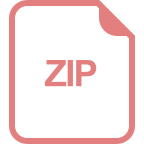
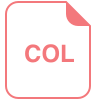
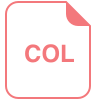
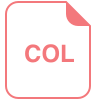
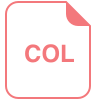



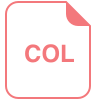
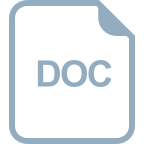