能显示实现的代码吗
时间: 2024-02-22 20:57:07 浏览: 52
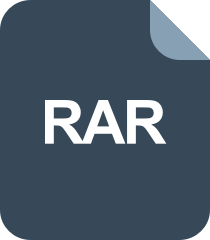
显示程序代码
以下是一个简单的 C# 代码示例,用于展示四象限图:
```csharp
using System;
using System.Windows.Forms;
using System.Windows.Forms.DataVisualization.Charting;
namespace FourQuadrantChart
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// 定义两列数据
double[] x = { 1, 2, 3, 4, 5 };
double[] y = { 2, 3, -1, -4, 5 };
// 添加数据到 Chart 控件中
chart1.Series.Clear();
chart1.Series.Add("Data");
chart1.Series["Data"].ChartType = SeriesChartType.Point;
for (int i = 0; i < x.Length; i++)
{
chart1.Series["Data"].Points.AddXY(x[i], y[i]);
}
// 设置散点图的样式和属性
chart1.Series["Data"].Color = System.Drawing.Color.Blue;
chart1.Series["Data"].MarkerStyle = MarkerStyle.Circle;
chart1.Series["Data"].MarkerSize = 10;
// 设置图表的属性
chart1.ChartAreas[0].AxisX.Minimum = 0;
chart1.ChartAreas[0].AxisX.Maximum = 6;
chart1.ChartAreas[0].AxisX.Interval = 1;
chart1.ChartAreas[0].AxisX.Title = "X Axis";
chart1.ChartAreas[0].AxisY.Minimum = -6;
chart1.ChartAreas[0].AxisY.Maximum = 6;
chart1.ChartAreas[0].AxisY.Interval = 1;
chart1.ChartAreas[0].AxisY.Title = "Y Axis";
chart1.ChartAreas[0].AxisX.MajorGrid.LineColor = System.Drawing.Color.LightGray;
chart1.ChartAreas[0].AxisY.MajorGrid.LineColor = System.Drawing.Color.LightGray;
// 添加一个按钮控件,用于计算和展示趋势线和交点位置
Button button1 = new Button();
button1.Text = "Calculate";
button1.Location = new System.Drawing.Point(10, 10);
button1.Click += new EventHandler(button1_Click);
this.Controls.Add(button1);
}
private void button1_Click(object sender, EventArgs e)
{
// 使用线性回归算法计算趋势线的斜率和截距
double[] x = { 1, 2, 3, 4, 5 };
double[] y = { 2, 3, -1, -4, 5 };
double sumX = 0, sumY = 0, sumXY = 0, sumX2 = 0;
for (int i = 0; i < x.Length; i++)
{
sumX += x[i];
sumY += y[i];
sumXY += x[i] * y[i];
sumX2 += x[i] * x[i];
}
double slope = (x.Length * sumXY - sumX * sumY) / (x.Length * sumX2 - sumX * sumX);
double intercept = (sumY - slope * sumX) / x.Length;
// 添加趋势线
chart1.Series.Add("TrendLine");
chart1.Series["TrendLine"].Color = System.Drawing.Color.Red;
chart1.Series["TrendLine"].ChartType = SeriesChartType.Line;
chart1.Series["TrendLine"].Points.AddXY(0, intercept);
chart1.Series["TrendLine"].Points.AddXY(6, slope * 6 + intercept);
chart1.Series["TrendLine"].ToolTip = "y = " + slope.ToString("0.000") + "x + " + intercept.ToString("0.000");
// 计算交点位置
double x0 = -intercept / slope;
double y0 = 0;
if (x0 > 0 && x0 < 6)
{
y0 = slope * x0 + intercept;
}
// 添加注释
chart1.Annotations.Clear();
chart1.Annotations.Add(new TextAnnotation
{
Text = "(" + x0.ToString("0.0") + ", " + y0.ToString("0.0") + ")",
X = x0,
Y = y0,
ForeColor = System.Drawing.Color.Red,
Font = new System.Drawing.Font("Arial", 10),
LineColor = System.Drawing.Color.Transparent
});
// 分割图表成四个象限
chart1.ChartAreas[0].AxisX.Crossing = y0;
chart1.ChartAreas[0].AxisY.Crossing = x0;
chart1.ChartAreas[0].AxisX.ArrowStyle = AxisArrowStyle.Triangle;
chart1.ChartAreas[0].AxisY.ArrowStyle = AxisArrowStyle.Triangle;
chart1.ChartAreas[0].AxisX.LineColor = System.Drawing.Color.Black;
chart1.ChartAreas[0].AxisY.LineColor = System.Drawing.Color.Black;
// 添加数据点
chart1.Series.Add("Data1");
chart1.Series["Data1"].ChartType = SeriesChartType.Point;
chart1.Series["Data1"].Color = System.Drawing.Color.Green;
chart1.Series["Data1"].MarkerStyle = MarkerStyle.Circle;
chart1.Series["Data1"].MarkerSize = 10;
chart1.Series.Add("Data2");
chart1.Series["Data2"].ChartType = SeriesChartType.Point;
chart1.Series["Data2"].Color = System.Drawing.Color.Orange;
chart1.Series["Data2"].MarkerStyle = MarkerStyle.Circle;
chart1.Series["Data2"].MarkerSize = 10;
for (int i = 0; i < x.Length; i++)
{
if (x[i] > x0 && y[i] > y0)
{
chart1.Series["Data1"].Points.AddXY(x[i], y[i]);
}
else if (x[i] > x0 && y[i] < y0)
{
chart1.Series["Data2"].Points.AddXY(x[i], y[i]);
}
else if (x[i] < x0 && y[i] < y0)
{
chart1.Series["Data1"].Points.AddXY(x[i], y[i]);
}
else if (x[i] < x0 && y[i] > y0)
{
chart1.Series["Data2"].Points.AddXY(x[i], y[i]);
}
}
}
}
}
```
以上代码实现了基本的四象限图的制作,可以根据实际需求进行调整和优化。
阅读全文
相关推荐
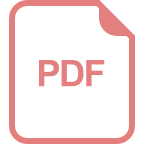
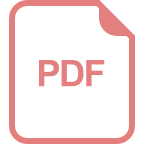
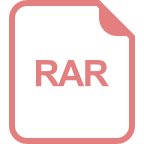
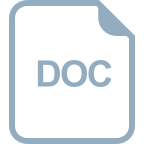
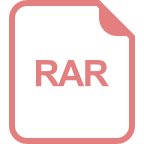
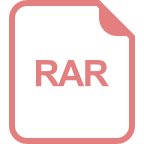
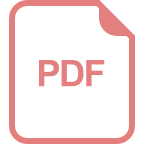
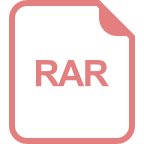
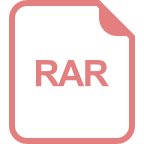
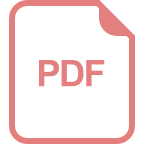
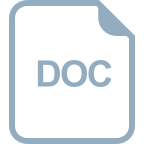