解释代码import java.io.IOException; import java.util.Arrays; import org.apache.hadoop.hbase.client.Mutation; import org.apache.hadoop.hbase.client.Put; import org.apache.hadoop.hbase.io.ImmutableBytesWritable; import org.apache.hadoop.hbase.mapreduce.TableReducer; import org.apache.hadoop.hbase.util.Bytes; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Reducer; public class mypreduce extends TableReducer<Text, Text, ImmutableBytesWritable> { @Override protected void reduce(Text k3, Iterable<Text> v3, Reducer<Text, Text, ImmutableBytesWritable, Mutation>.Context context) throws IOException, InterruptedException { int avg=0; for(Text i:v3){ System.out.println(k3.toString()+","+i.toString()); int sum=0; int count=0; String[] scores=i.toString().split(" "); System.out.println(Arrays.toString(scores)); //逐个将每个学生的成绩分数相加 for(String score:scores){ sum=sum+Integer.parseInt(score); count++; //System.out.println("reduce52"); } System.out.println(sum); //计算每个学生的平均分数 avg=sum/count; System.out.println(avg); } //将计算出的每个学生的平均分数添加到HBase表格中 Put put=new Put(Bytes.toBytes(k3.toString())); put.addColumn(Bytes.toBytes("avg"),null,Bytes.toBytes(String.valueOf(avg))); context.write(new ImmutableBytesWritable(Bytes.toBytes(k3.toString())), put); } }
时间: 2024-04-28 14:20:34 浏览: 8
这是一个 Hadoop MapReduce 的 Reduce 阶段的代码,用于将一个学生的多次考试成绩求平均分并写入 HBase 表格中。具体来说,这段代码继承自 HBase 的 TableReducer 类,输入的键值对类型为 Text 和 Text,输出的键值对类型为 ImmutableBytesWritable 和 Mutation。在 reduce() 方法中,首先遍历每个学生的成绩列表,将每个学生的成绩相加并计算平均分,然后将计算出的平均分作为值写入 HBase 表格中。其中,Put 对象表示一行数据,包含了行键、列族、列名和列值等信息。ImmutableBytesWritable 类表示 HBase 表格中的行键,Mutation 是一个抽象类,其子类 Put 表示要写入一行数据。
相关问题
import java.io.IOException; import java.util.ArrayList;
你好!这不是一个问题,而是一段 Java 代码。这段代码导入了两个包,一个是 Java IO 包,另一个是 Java 集合框架中的 ArrayList 类。其中,Java IO 包提供了输入输出相关的类和接口,而 ArrayList 是一种可以动态增长的数组,可以方便地进行元素的添加、删除和遍历。你有什么关于这些包的问题吗?
``` import java.io.IOException;```解释意思
这是Java编程语言中的一个import语句,它表示导入java.io包中的IOException类。IOException类是Java中处理输入/输出异常的一个标准类,它提供了一组方法来处理IO异常,如文件读写、网络通信等。当Java程序在读写文件或者进行网络通信时,如果出现IO异常,就可以使用IOException类来捕获并处理这些异常。
相关推荐
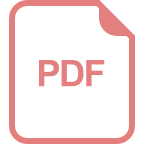
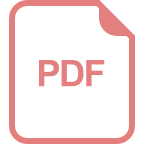
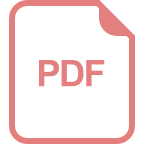
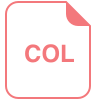












