excel完整的导出pdf java
时间: 2023-07-12 21:04:30 浏览: 164
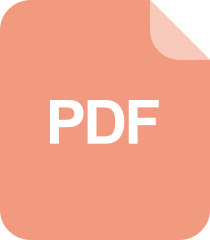
java导出Excel文件.pdf
要将Excel文件导出为PDF,您可以使用Apache POI和iText库来完成Java代码。下面是一个简单的示例代码,它将Excel文件转换为PDF文件:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfWriter;
public class ExcelToPdfConverter {
public static void main(String[] args) throws IOException {
String excelFilePath = "path/to/excel/file.xlsx";
String pdfFilePath = "path/to/pdf/file.pdf";
Workbook workbook = getWorkbook(excelFilePath);
OutputStream out = new FileOutputStream(pdfFilePath);
Document document = new Document();
PdfWriter.getInstance(document, out);
document.open();
// Loop through each sheet in the workbook
for (int i = 0; i < workbook.getNumberOfSheets(); i++) {
// Get the sheet
org.apache.poi.ss.usermodel.Sheet sheet = workbook.getSheetAt(i);
// Loop through each row in the sheet
for (int j = 0; j <= sheet.getLastRowNum(); j++) {
// Get the row
org.apache.poi.ss.usermodel.Row row = sheet.getRow(j);
// Loop through each cell in the row
if (row != null) {
for (int k = 0; k < row.getLastCellNum(); k++) {
// Get the cell
org.apache.poi.ss.usermodel.Cell cell = row.getCell(k);
// Write the cell value to the PDF document
document.add(new com.itextpdf.text.Paragraph(cell.getStringCellValue()));
}
}
}
}
document.close();
out.close();
System.out.println("Excel file converted to PDF successfully!");
}
private static Workbook getWorkbook(String excelFilePath) {
Workbook workbook = null;
try {
if (excelFilePath.endsWith("xlsx")) {
workbook = new XSSFWorkbook(excelFilePath);
} else if (excelFilePath.endsWith("xls")) {
workbook = new HSSFWorkbook(excelFilePath);
}
} catch (IOException e) {
e.printStackTrace();
}
return workbook;
}
}
```
请注意,此代码仅处理单元格中的文本内容,并且仅支持.xls和.xlsx格式的Excel文件。您可以根据需要进行修改。
阅读全文
相关推荐
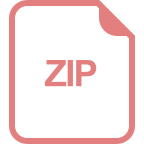
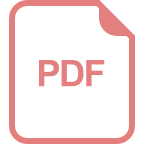
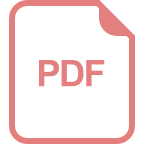
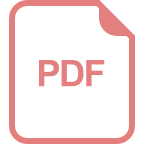
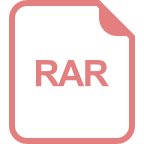
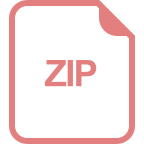
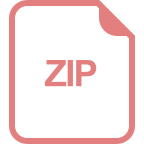
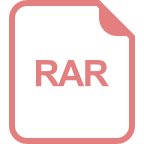
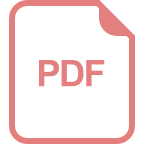
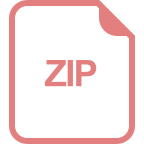
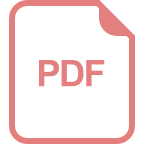
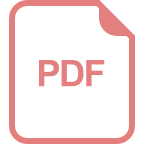
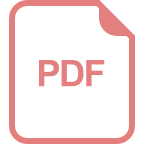
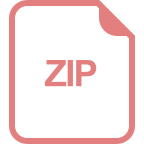
