用python写一个完整的爬虫代码,爬虫可以爬取微博的ID、图片、内容、点赞数、评论数,并将ID、内容、点赞数、评论数保存为xls格式,同一个ID下的图片保存到同一个文件夹里
时间: 2024-06-09 07:07:50 浏览: 23
好的,以下是一个简单的微博爬虫代码,需要使用到requests、beautifulsoup4、lxml、openpyxl、os等库:
```python
import requests
from bs4 import BeautifulSoup
import lxml
import openpyxl
import os
# 爬取微博
def get_weibo():
# 定义要爬取的微博页面url
url = 'https://weibo.com/u/1234567890?is_all=1'
# 添加请求头,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 发送请求
response = requests.get(url, headers=headers)
# 解析网页内容
soup = BeautifulSoup(response.text, 'lxml')
# 提取微博信息
weibo_list = []
for item in soup.find_all('div', class_='WB_detail'):
try:
# 获取微博ID
weibo_id = item.find('a', class_='W_texta W_fb').get('name')
# 获取微博内容
weibo_content = item.find('div', class_='WB_text W_f14').text.strip()
# 获取微博点赞数
weibo_like = item.find('span', class_='line S_line1').find_all('em')[0].text
# 获取微博评论数
weibo_comment = item.find('span', class_='line S_line1').find_all('em')[1].text
# 获取微博图片
weibo_img_list = []
for img in item.find_all('img', class_='bigcursor'):
weibo_img_list.append(img.get('src'))
# 将微博信息添加到列表中
weibo_list.append([weibo_id, weibo_content, weibo_like, weibo_comment, weibo_img_list])
except:
pass
return weibo_list
# 将微博信息保存为xls格式
def save_to_excel(weibo_list):
# 创建工作簿
wb = openpyxl.Workbook()
# 创建工作表
ws = wb.active
# 添加表头
ws.append(['微博ID', '微博内容', '点赞数', '评论数'])
# 遍历微博列表,写入数据
for weibo in weibo_list:
ws.append(weibo[0:4])
# 保存工作簿
wb.save('weibo.xlsx')
# 下载微博图片
def download_images(weibo_list):
# 创建目录
if not os.path.exists('weibo_images'):
os.mkdir('weibo_images')
# 遍历微博列表,下载图片
for weibo in weibo_list:
# 获取微博ID
weibo_id = weibo[0]
# 创建微博图片目录
weibo_img_path = 'weibo_images/' + str(weibo_id)
if not os.path.exists(weibo_img_path):
os.mkdir(weibo_img_path)
# 下载图片
for i, img_url in enumerate(weibo[4]):
img_path = weibo_img_path + '/' + str(i) + '.jpg'
try:
response = requests.get(img_url)
with open(img_path, 'wb') as f:
f.write(response.content)
except:
pass
if __name__ == '__main__':
# 爬取微博信息
weibo_list = get_weibo()
# 保存微博信息为xls格式
save_to_excel(weibo_list)
# 下载微博图片
download_images(weibo_list)
```
需要注意的是,此代码仅供学习参考,爬虫行为需要遵守相关法律法规,不得用于非法用途。
相关推荐
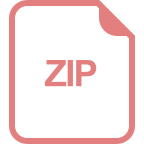
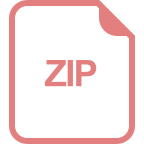









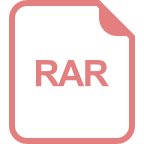