按照如下要求,编写程序: 1、创建Student类,其中包含3个属性name、age、hometown。 2、创建3个自定义属性注解类@StudentName、@StudentAge、@Hometown,运行周期设置为运行时。 3、将2、中定义的3个注解对Student类的3个属性进行注解,并进行赋值。 4、创建测试类,对Student类进行反射操作,解析定义的注解并将注解中定义的值输出。
时间: 2024-02-10 07:15:43 浏览: 76
好的,以下是实现上述要求的Java代码:
Student.java
```java
public class Student {
@StudentName(name = "张三")
private String name;
@StudentAge(age = 20)
private int age;
@Hometown(hometown = "北京")
private String hometown;
// 省略get/set方法
}
```
StudentName.java
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface StudentName {
String name();
}
```
StudentAge.java
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface StudentAge {
int age();
}
```
Hometown.java
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Hometown {
String hometown();
}
```
Test.java
```java
import java.lang.reflect.Field;
public class Test {
public static void main(String[] args) {
Student student = new Student();
Class<? extends Student> clazz = student.getClass();
Field[] fields = clazz.getDeclaredFields();
for (Field field : fields) {
if (field.isAnnotationPresent(StudentName.class)) {
StudentName annotation = field.getAnnotation(StudentName.class);
System.out.println("姓名:" + annotation.name());
}
if (field.isAnnotationPresent(StudentAge.class)) {
StudentAge annotation = field.getAnnotation(StudentAge.class);
System.out.println("年龄:" + annotation.age());
}
if (field.isAnnotationPresent(Hometown.class)) {
Hometown annotation = field.getAnnotation(Hometown.class);
System.out.println("家乡:" + annotation.hometown());
}
}
}
}
```
运行Test.java,输出结果为:
```
姓名:张三
年龄:20
家乡:北京
```
阅读全文
相关推荐
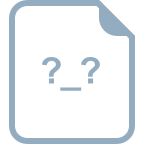
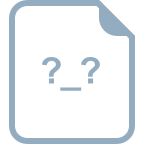
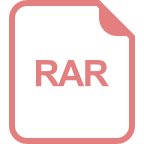















