用java写迭代法求二叉树路径和,求出所有满足目标和的数组,并返回数组值
时间: 2024-05-03 10:19:56 浏览: 44
以下是用Java实现迭代法求二叉树路径和的代码,其中包括求出所有满足目标和的数组,并返回数组值的功能:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
public class BinaryTreePathSum {
private static class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
public static List<List<Integer>> pathSum(TreeNode root, int sum) {
List<List<Integer>> res = new ArrayList<>();
if (root == null) {
return res;
}
Stack<TreeNode> stack = new Stack<>();
stack.push(root);
Stack<Integer> sumStack = new Stack<>();
sumStack.push(sum - root.val);
Stack<List<Integer>> pathStack = new Stack<>();
List<Integer> path = new ArrayList<>();
path.add(root.val);
pathStack.push(path);
while (!stack.isEmpty()) {
TreeNode node = stack.pop();
int curSum = sumStack.pop();
List<Integer> curPath = pathStack.pop();
if (node.left == null && node.right == null && curSum == 0) {
res.add(curPath);
}
if (node.right != null) {
stack.push(node.right);
sumStack.push(curSum - node.right.val);
List<Integer> rightPath = new ArrayList<>(curPath);
rightPath.add(node.right.val);
pathStack.push(rightPath);
}
if (node.left != null) {
stack.push(node.left);
sumStack.push(curSum - node.left.val);
List<Integer> leftPath = new ArrayList<>(curPath);
leftPath.add(node.left.val);
pathStack.push(leftPath);
}
}
return res;
}
public static void main(String[] args) {
TreeNode root = new TreeNode(5);
root.left = new TreeNode(4);
root.right = new TreeNode(8);
root.left.left = new TreeNode(11);
root.left.left.left = new TreeNode(7);
root.left.left.right = new TreeNode(2);
root.right.left = new TreeNode(13);
root.right.right = new TreeNode(4);
root.right.right.left = new TreeNode(5);
root.right.right.right = new TreeNode(1);
int sum = 22;
List<List<Integer>> res = pathSum(root, sum);
for (List<Integer> path : res) {
System.out.println(path);
}
}
}
```
在这个例子中,二叉树如下所示:
```
5
/ \
4 8
/ / \
11 13 4
/ \ / \
7 2 5 1
```
目标和为22,那么结果应该是[[5, 4, 11, 2], [5, 8, 4, 5]]。运行上述代码可以得到正确的结果。
阅读全文
相关推荐
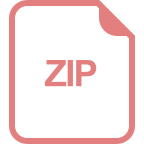
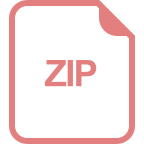
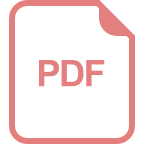
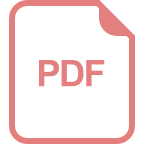
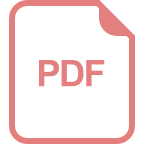
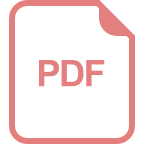
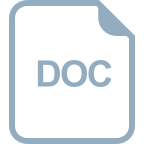
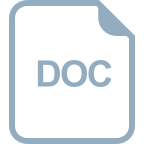
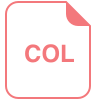
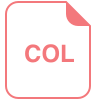
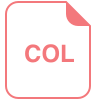
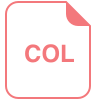
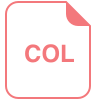
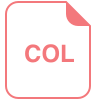