递归算法深度解析:Java中的分治法和回溯法实例
发布时间: 2024-08-29 11:34:27 阅读量: 37 订阅数: 26 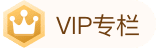
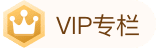
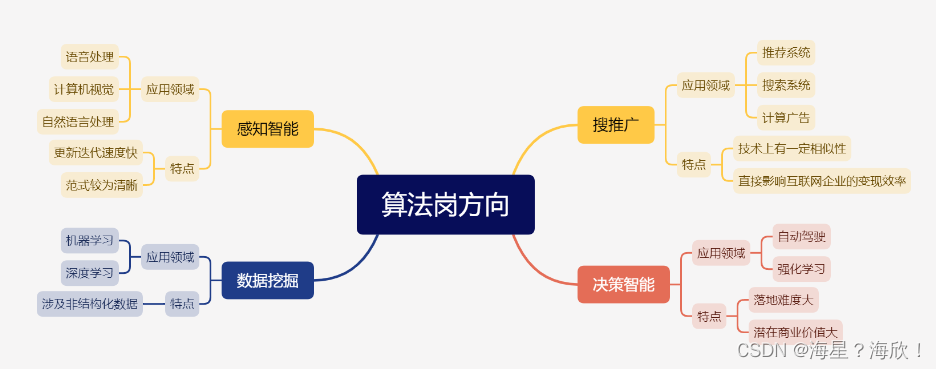
# 1. 递归算法概述
递归算法是计算机科学领域中一种极为重要的算法设计思想,它的核心在于函数自我调用。递归通常用于解决可以分解为相似子问题的问题,其基本思想是将原问题划分成规模较小、易于解决的子问题,并通过递归调用自身以求解子问题,最终合并子问题的解以得出原问题的解。递归算法简洁且易于理解,但在实际应用中,它也有可能导致效率低下和栈溢出的问题。
递归函数的两个核心要素是:
1. 基线条件(Base Case):是递归停止的条件,防止无限递归发生。
2. 递归条件(Recursive Case):是函数自我调用的逻辑。
递归算法的例子非常丰富,如排序算法中的快速排序和归并排序,树结构的遍历等,都是递归的经典应用。随着学习的深入,我们会探索分治法、回溯法等递归策略的高级应用和优化技巧。
# 2. ```
# 第二章:分治法详解
## 2.1 分治法的基本概念和原理
### 2.1.1 分治法定义及其在递归中的作用
分治法(Divide and Conquer)是一种在计算机科学中广泛使用的算法设计范式。它的核心思想是将一个难以直接解决的大问题分解为一些规模较小的相同问题,递归解决这些子问题,然后合并这些子问题的解以得到原问题的解。分治法通常有三个步骤:分解(Divide)、解决(Conquer)和合并(Combine)。
在递归算法中,分治法的作用尤为显著。递归算法是分治法的一个典型应用场景,它通过函数自己调用自己来解决问题,其背后往往是以分治的思想来设计的。使用分治法可以简化问题的解决步骤,通过将问题分割成更小的单元,递归地进行求解,最后将子问题的解进行合并,从而得到原问题的解。
### 2.1.2 分治法的算法框架和应用领域
分治法的算法框架可表示为以下伪代码:
```plaintext
Divide-Conquer(P)
if |P| <= n0
return base case solution of P
divide P into k subsets P1, P2, ..., Pk of size n/n0
for i = 1 to k
Pi' <- Divide-Conquer(Pi)
return Combine(P1', P2', ..., Pk')
```
其中,`n0` 是划分问题的一个阈值,`base case` 是可以直接解决的最小问题规模。该框架说明了分治法的三个基本步骤:
1. **分解**:将原问题分解成规模更小的问题。
2. **解决**:递归求解各个子问题。如果子问题足够小,则直接解决。
3. **合并**:将子问题的解合并成原问题的解。
分治法的应用领域非常广泛,包括但不限于:
- **排序算法**:如归并排序、快速排序。
- **搜索算法**:如二分搜索。
- **矩阵运算**:如Strassen矩阵乘法。
- **数值分析**:如多项式的快速傅里叶变换(FFT)。
- **图算法**:如最近点对问题。
## 2.2 分治法在Java中的实现技巧
### 2.2.1 分治法算法模板和案例分析
在Java中,分治法通常通过递归函数实现。下面给出一个分治法的通用模板:
```java
public class DivideAndConquer {
public static int[] divideAndConquer(int[] input, int left, int right) {
if (left == right) {
// Base case: 如果只有一个元素,则直接返回结果
return new int[]{input[left]};
}
// 分解:将问题划分为较小的子问题
int mid = (left + right) / 2;
int[] leftResult = divideAndConquer(input, left, mid);
int[] rightResult = divideAndConquer(input, mid + 1, right);
// 解决:合并子问题的解
return merge(leftResult, rightResult);
}
private static int[] merge(int[] leftResult, int[] rightResult) {
// 该函数用于合并左右子问题的结果
// 具体合并逻辑依据具体问题而定
return null;
}
public static void main(String[] args) {
int[] array = {1, 3, 5, 7, 9, 11};
int[] result = divideAndConquer(array, 0, array.length - 1);
// 输出结果...
}
}
```
在模板中,`divideAndConquer` 函数负责将问题分解并递归求解子问题,`merge` 函数用于合并解。
### 2.2.2 分治法与动态规划的比较
分治法与动态规划都是通过分解问题来达到简化问题解决难度的方法,但它们在解决问题的方式和适用性上有很大的区别:
- **适用性**:分治法适用于子问题相互独立的情况,动态规划则适用于子问题重叠,即相同子问题需要多次求解的情况。
- **记忆化**:动态规划通常利用记忆化(如使用数组存储子问题的解)来避免重复计算,而分治法的递归过程中不一定存储中间结果。
- **性能**:动态规划往往能够提供更好的性能,因为其优化了子问题的求解次数。
## 2.3 分治法经典问题实例
### 2.3.1 快速排序算法的分治法实现
快速排序是一种典型的分治法排序算法。其基本思想是选择一个基准值(pivot),通过一趟排序将待排序记录分割成独立的两部分,其中一部分记录的值均比基准值小,另一部分记录的值比基准值大,然后分别对这两部分记录继续进行排序,以达到整个序列有序。
快速排序的分治法伪代码如下:
```plaintext
QuickSort(A, low, high)
if low < high then
pivot位置 <- Partition(A, low, high)
QuickSort(A, low, pivot位置 - 1)
QuickSort(A, pivot位置 + 1, high)
```
其中,`Partition` 函数负责根据基准值将数组分割成两部分,并返回基准值的位置。
### 2.3.2 归并排序算法的分治法实现
归并排序同样是一个利用分治法思想的排序算法。它将已有序的子序列合并,得到完全有序的序列;即先使每个子序列有序,再使子序列段间有序。
归并排序的分治法伪代码如下:
```plaintext
MergeSort(A, p, r)
if p < r then
q <- (p + r) / 2
MergeSort(A, p, q)
MergeSort(A, q + 1, r)
Merge(A, p, q, r)
```
其中,`Merge` 函数负责将两个有序数组合并成一个有序数组。
### 分析
分治法为解决计算机科学中的复杂问题提供了一个强有力的工具。通过递归的方式,分治法能够将大问题逐步简化为小问题,最终得到问题的解决方案。在实际编程中,掌握了分治法的设计思想和实现技巧,就能更好地解决诸如排序、搜索、图论等领域的问题。
分治法的实现要点在于正确地分解问题、递归解决子问题以及有效地合并子问题的解。在某些情况下,分治法可能需要和其他算法设计方法相结合,比如动态规划、贪心算法等,以达到更优的性能表现。
```
# 3. 回溯法详解
回溯法是一种基于试错的搜索算法,通过尝试所有可能的候选解来找出所有的解。如果候选解被确认不是一个有效的解(或者至少不是最后一个解),回溯算法会丢弃该解,即回溯并且在剩余的解空间中继续尝试。由于回溯法采用了递归的形式来遍历问题的解空间,因此它也可以归类为一种递归算法。
### 3.1 回溯法的基本概念和原理
#### 3.1.1 回溯法定义及其与分治法的区别
回溯法与分治法类似,都采用递归思想,但它们的应用场景和解决问题的方式有所不同。回溯法常用于求解约束满足问题,如八皇后问题、图的着色、旅行商问题等,这些问题通常没有明显的子结构划分,或者子结构之间的联系较为复杂。
回溯法的特点是“走不通就回头”,在遇到不满足条件的情况时,会回退到上一步,尝试其他可能性。分治法通常将问题划分成相互独立的子问题,而回溯法则是在同一问题空间内进行试探,它所面临的子问题通常是原问题的更小子集。
#### 3.1.2 回溯法的算法框架和搜索策略
回溯法的基本算法框架如下:
1. 针对每一项决策,尝试所有可能的选项。
2. 判断当前选项是否满足问题的约束条件。
3. 如果当前选项不能导致问题的解,则撤销该决策(回溯)。
4. 如果当前选项可以导致问题的解,则记录该解。
5. 继续尝试下一个决策,直到所
0
0
相关推荐
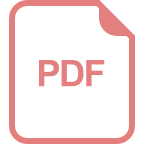
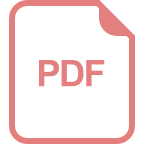
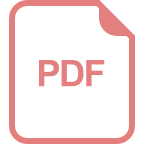





