一个简单的web前端打地鼠小游戏
时间: 2023-09-17 08:15:08 浏览: 67
好的,下面是一个简单的web前端打地鼠小游戏的实现,使用HTML、CSS和JavaScript编写。
HTML部分:
```html
<!DOCTYPE html>
<html>
<head>
<title>打地鼠小游戏</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<h1>打地鼠小游戏</h1>
<div class="game-container">
<div class="hole hole1"></div>
<div class="hole hole2"></div>
<div class="hole hole3"></div>
<div class="hole hole4"></div>
<div class="hole hole5"></div>
<div class="hole hole6"></div>
<div class="score">得分:<span id="score">0</span></div>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS部分:
```css
.game-container {
margin: 0 auto;
width: 500px;
height: 400px;
position: relative;
}
.hole {
width: 100px;
height: 100px;
background-image: url("hole.png");
background-size: 100% 100%;
position: absolute;
top: 50px;
left: 50px;
cursor: pointer;
display: none;
}
.hole1 {
top: 50px;
left: 50px;
}
.hole2 {
top: 50px;
left: 175px;
}
.hole3 {
top: 50px;
left: 300px;
}
.hole4 {
top: 175px;
left: 50px;
}
.hole5 {
top: 175px;
left: 175px;
}
.hole6 {
top: 175px;
left: 300px;
}
.score {
font-size: 24px;
font-weight: bold;
text-align: center;
margin-top: 20px;
}
.mole {
width: 100px;
height: 100px;
background-image: url("mole.png");
background-size: 100% 100%;
position: absolute;
top: 50px;
left: 50px;
display: none;
cursor: pointer;
animation: mole-up 0.5s ease-in-out;
}
@keyframes mole-up {
0% {
transform: translateY(0);
}
50% {
transform: translateY(-50px);
}
100% {
transform: translateY(0);
}
}
```
JavaScript部分:
```javascript
var score = 0;
var timeLeft = 30;
var timerId = null;
var moleTimerId = null;
function startGame() {
score = 0;
timeLeft = 30;
document.getElementById("score").innerHTML = score;
document.getElementById("time-left").innerHTML = timeLeft;
document.getElementById("start-button").disabled = true;
timerId = setInterval(timerTick, 1000);
moleTimerId = setInterval(showMole, 1000);
}
function timerTick() {
timeLeft--;
document.getElementById("time-left").innerHTML = timeLeft;
if (timeLeft === 0) {
clearInterval(timerId);
clearInterval(moleTimerId);
alert("游戏结束,你的得分是:" + score);
document.getElementById("start-button").disabled = false;
}
}
function showMole() {
var holes = document.getElementsByClassName("hole");
var randomIndex = Math.floor(Math.random() * holes.length);
var hole = holes[randomIndex];
var mole = hole.nextElementSibling;
hole.style.display = "block";
mole.style.display = "block";
setTimeout(hideMole, 1000, hole, mole);
}
function hideMole(hole, mole) {
hole.style.display = "none";
mole.style.display = "none";
}
function hitMole(event) {
if (event.target.classList.contains("mole")) {
score++;
document.getElementById("score").innerHTML = score;
event.target.style.display = "none";
}
}
var holes = document.getElementsByClassName("hole");
for (var i = 0; i < holes.length; i++) {
holes[i].addEventListener("click", hitMole);
}
```
这个小游戏的实现方式是:在页面上创建6个“洞”,然后每秒随机在其中一个洞里“钻出”一个地鼠,玩家需要在1秒内点击地鼠,击中后得分加1。游戏时长为30秒,时间到后弹出得分提示框。
相关推荐
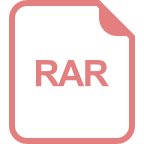
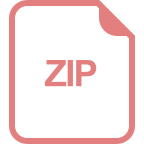














