scala string类型转成日期格式
时间: 2023-10-17 07:10:56 浏览: 180
你可以使用Java中的SimpleDateFormat类来将Scala中的字符串转换为日期格式。以下是一个示例代码:
```scala
import java.text.SimpleDateFormat
import java.util.Date
val dateString = "2021-10-15"
val dateFormat = new SimpleDateFormat("yyyy-MM-dd")
val date = dateFormat.parse(dateString)
```
在上面的代码中,我们首先定义一个字符串`dateString`,它包含日期信息。然后我们创建了一个`SimpleDateFormat`对象,并使用`yyyy-MM-dd`格式化字符串表示日期。最后,我们使用`parse`方法将字符串转换为`Date`类型。
需要注意的是,`parse`方法可能会抛出`ParseException`异常,因此我们需要使用`try-catch`语句来捕获它。
相关问题
scala 的string.format 用法
在 Scala 中,可以使用 `format()` 方法来格式化字符串。该方法与 Java 中的 `String.format()` 方法类似。
以下是 `format()` 方法的基本用法:
```scala
val str = "Hello, %s!".format("Scala")
println(str) // 输出:Hello, Scala!
```
在上面的例子中,`%s` 是格式化字符串中的占位符,它将被后面的参数 `"Scala"` 替换。
除了 `%s` 之外,还有其他类型的占位符可以使用。以下是常见的占位符类型及其用法:
| 占位符 | 类型 | 示例 |
|--------|------------|--------------------------|
| `%s` | 字符串 | `"Hello, %s!".format("world")` → `"Hello, world!"` |
| `%d` | 整数 | `"I have %d apples".format(3)` → `"I have 3 apples"` |
| `%f` | 浮点数 | `"Pi is approximately %f".format(3.14159)` → `"Pi is approximately 3.141590"` |
| `%c` | 字符 | `"The first letter of the alphabet is %c".format('A')` → `"The first letter of the alphabet is A"` |
| `%b` | 布尔值 | `"It is %b that 1 + 1 = 3".format(false)` → `"It is false that 1 + 1 = 3"` |
| `%x` | 十六进制数 | `"The number 255 in hex is %x".format(255)` → `"The number 255 in hex is ff"` |
除了基本类型之外,还可以使用额外的占位符来指定更复杂的格式,如日期和时间等。例如:
```scala
import java.time.LocalDateTime
val now = LocalDateTime.now()
println("Current time: %tT".format(now)) // 输出:Current time: 16:20:30
```
这里的 `%tT` 是时间格式的占位符。有关更多占位符类型及其用法,请参阅 Scala 官方文档。
scala spark 将 dwd 库中表的数据关联到 dws 库中 table12 的分区表,分区字段为 etl_date,类型为 String,且值为当前比赛日的前一天日期(分区字段格式 为 yyyyMMdd),并添加 dws_insert_user、dws_insert_time、dws_modify_user、 dws_modify_time 四列,其中 dws_insert_user、dws_modify_user 均填写 “user1”,dws_insert_time、dws_modify_time 均填写当前操作时间(年 月日必须是今天,时分秒只需在比赛时间范围内即可),并进行数据类型转 换
假设我们的dwd库中有一个表叫做dwd_table,我们需要将其关联到dws库中的table12分区表中,可以按照下面的步骤进行操作:
1. 创建dws库中的table12分区表,并定义分区字段etl_date,创建四个额外的列dws_insert_user、dws_insert_time、dws_modify_user、dws_modify_time:
```scala
spark.sql("CREATE TABLE dws.table12 (col1 String, col2 Int, etl_date String) PARTITIONED BY (etl_date String) " +
"TBLPROPERTIES('parquet.compress'='SNAPPY', 'parquet.compression.codec'='snappy')")
spark.sql("ALTER TABLE dws.table12 ADD COLUMNS (dws_insert_user String, dws_insert_time Timestamp, " +
"dws_modify_user String, dws_modify_time Timestamp)")
```
2. 获取比赛日的前一天日期,并将其转换为字符串格式:
```scala
import java.time.LocalDate
import java.time.format.DateTimeFormatter
val dtFormatter = DateTimeFormatter.ofPattern("yyyyMMdd")
val etl_date = dtFormatter.format(LocalDate.now().minusDays(1))
```
3. 将dwd_table中的数据关联到dws库中的table12分区表中,分区字段为etl_date,同时添加四个额外的列:
```scala
val insert_user = "user1"
val modify_user = "user1"
val insert_time = java.sql.Timestamp.valueOf(java.time.LocalDateTime.now())
val modify_time = java.sql.Timestamp.valueOf(java.time.LocalDateTime.now())
spark.sql(s"INSERT INTO dws.table12 PARTITION(etl_date='$etl_date')" +
s"SELECT col1, col2, '$etl_date', '$insert_user', '$insert_time', '$modify_user', '$modify_time' " +
"FROM dwd.dwd_table")
```
4. 对添加的四个列进行数据类型转换:
```scala
spark.sql("ALTER TABLE dws.table12 " +
"CHANGE COLUMN dws_insert_time dws_insert_time Timestamp " +
"CHANGE COLUMN dws_modify_time dws_modify_time Timestamp")
```
这样,我们就完成了将dwd库中表的数据关联到dws库中table12的分区表中,并添加四个额外列的操作。
阅读全文
相关推荐
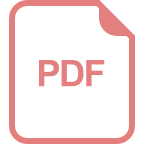
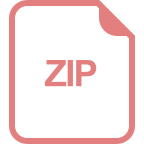
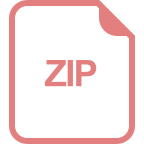














