用c++写一个识别灯条并输出距离的代码
时间: 2023-02-11 10:59:56 浏览: 138
这里是一个简单的例子,它使用 OpenCV 库来识别灯条并计算距离。
```
#include <opencv2/opencv.hpp>
#include <iostream>
int main() {
cv::VideoCapture cap(0); // 打开摄像头
if (!cap.isOpened()) {
std::cout << "摄像头打开失败" << std::endl;
return -1;
}
while (true) {
cv::Mat frame;
cap >> frame; // 获取当前帧
// 灰度化、高斯模糊、二值化
cv::Mat gray, blur, threshold;
cvtColor(frame, gray, cv::COLOR_BGR2GRAY);
GaussianBlur(gray, blur, cv::Size(5, 5), 0);
threshold(blur, threshold, 100, 255, cv::THRESH_BINARY);
// 查找轮廓
std::vector<std::vector<cv::Point>> contours;
cv::findContours(threshold, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
// 遍历每个轮廓
for (int i = 0; i < contours.size(); i++) {
// 筛选符合条件的轮廓
if (cv::contourArea(contours[i]) > 50) {
// 计算轮廓的最小外接矩形
cv::Rect rect = cv::boundingRect(contours[i]);
cv::rectangle(frame, rect, cv::Scalar(0, 0, 255), 2);
// 计算距离(假设灯条高度为 h,摄像头与灯条的距离为 d)
double h = rect.height;
double d = (knownHeight * focalLength) / h;
std::cout << "距离: " << d << " 厘米" << std::endl;
}
}
cv::imshow("frame", frame); // 显示当前帧
if (cv::waitKey(30) >= 0) {
break;
}
}
return 0;
}
```
请注意,这只是一个简单的例子,实际使用中可能
阅读全文
相关推荐
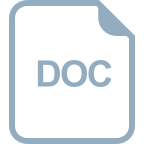
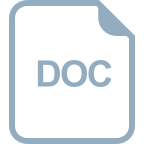
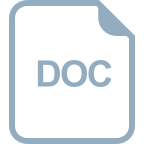
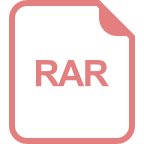
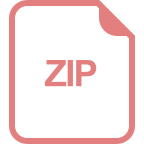
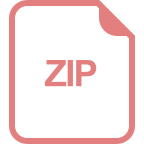
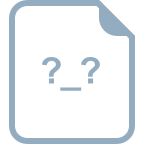
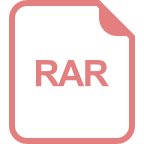
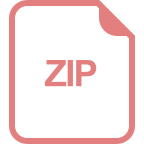
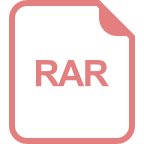
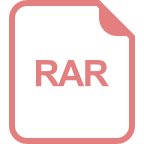
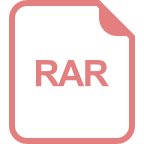
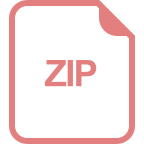