public class DatabaseManage { private Context mContext = null; private SQLiteDatabase mSQLiteDatabase = null;//用于操作数据库的对象 private DatabaseHelper dh = null;//用于创建数据库的对象 private String dbName = "notepad.db"; private int dbVersion = 1; public DatabaseManage(Context context){ mContext = context; } /** * 打开数据库 */ public void open(){ try{ dh = new DatabaseHelper(mContext, dbName, null, dbVersion); if(dh == null){ Log.v("msg", "is null"); return ; } mSQLiteDatabase = dh.getWritableDatabase(); }catch(SQLiteException se){ se.printStackTrace(); } } /** * 关闭数据库 */ public void close(){ mSQLiteDatabase.close(); dh.close(); } //获取列表 public Cursor selectAll(){ Cursor cursor = null; try{ String sql = "select * from record"; cursor = mSQLiteDatabase.rawQuery(sql, null); }catch(Exception ex){ ex.printStackTrace(); cursor = null; } return cursor; } public Cursor selectById(int id){ Cursor cursor = null; try{ String sql = "select * from record where _id='" + id +"'"; cursor = mSQLiteDatabase.rawQuery(sql, null); }catch(Exception ex){ ex.printStackTrace(); cursor = null; } return cursor; } //插入数据 public long insert(String title, String content){ long datetime = System.currentTimeMillis(); long l = -1; try{ ContentValues cv = new ContentValues(); cv.put("title", title); cv.put("content", content); cv.put("time", datetime); l = mSQLiteDatabase.insert("record", null, cv); }catch(Exception ex){ ex.printStackTrace(); l = -1; } return l; } //删除数据 public int delete(long id){ int affect = 0; try{ affect = mSQLiteDatabase.delete("record", "_id=?", new String[]{id+""}); }catch(Exception ex){ ex.printStackTrace(); affect = -1; } return affect; } //修改数据 public int update(int id, String title, String content){ int affect = 0; try{ ContentValues cv = new ContentValues(); cv.put("title", title); cv.put("content", content); String w[] = {id+""}; affect = mSQLiteDatabase.update("record", cv, "_id=?", w); }catch(Exception ex){ ex.printStackTrace(); affect = -1; } return affect; } }这段代码的作用
时间: 2024-02-02 14:03:29 浏览: 122
这段代码是一个用于操作 SQLite 数据库的工具类。它包含了数据库的打开和关闭、查询、插入、删除和更新数据等操作。具体实现如下:
在构造函数中,传入上下文信息。
open() 方法用于打开数据库,创建一个 DatabaseHelper 对象,并调用 getWritableDatabase() 方法获取可写的数据库对象。
close() 方法用于关闭数据库。
selectAll() 方法查询所有数据,并返回一个 Cursor 对象。
selectById(int id) 方法根据给定的 id 查询数据,并返回一个 Cursor 对象。
insert(String title, String content) 方法插入一条记录,并返回插入的记录的行号。
delete(long id) 方法根据给定的 id 删除一条记录,并返回影响的行数。
update(int id, String title, String content) 方法根据给定的 id 更新一条记录,并返回影响的行数。
这个工具类用于封装常用的数据库操作,便于在应用中使用。
相关问题
package com.example.homework6_1; import android.content.Context; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteDatabase.CursorFactory; import android.database.sqlite.SQLiteOpenHelper; public class DatabaseHelper extends SQLiteOpenHelper { private String tableName = "record"; private Context mContext = null; private String sql = "create table if not exists " + tableName + "(_id integer primary key autoincrement, " + "title varchar," + "content text," + "time varchar)"; public DatabaseHelper(Context context, String name, CursorFactory factory, int version) { super(context, name, factory, version); } @Override public void onCreate(SQLiteDatabase db) { //创建表 db.execSQL(sql); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { } }解释
这段代码是一个在 Android 平台上使用 SQLite 数据库的帮助类。该类继承于 SQLiteOpenHelper 类,用于创建和升级数据库。它包含一个数据库表的表名、上下文、创建表的 SQL 语句等信息。
在构造函数中,传入数据库的名称、工厂和版本号。在 onCreate() 方法中,执行 SQL 语句创建表。在 onUpgrade() 方法中,用于升级数据库版本,但这个方法没有实现任何操作。
public class MemoAdapter extends RecyclerView.Adapter<MemoAdapter.ViewHolder> { private Context mcontext; private List<MemoBean> arr1; private MyDbHelper mhelper1; private SQLiteDatabase db; public MemoAdapter(Context mcontext, List<MemoBean> arr1) { this.mcontext = mcontext; this.arr1 = arr1; }
这是 MemoAdapter 类的一部分代码,它是一个适配器类,用于将 MemoBean 对象列表 arr1 绑定到 RecyclerView 中。该适配器包含一个上下文对象 mcontext、一个 MemoBean 对象列表 arr1、一个 MyDbHelper 对象 mhelper1 和一个 SQLiteDatabase 对象 db。
构造函数 MemoAdapter(Context mcontext, List<MemoBean> arr1) 接收一个上下文对象和一个 MemoBean 对象列表作为参数,并将它们分别保存在成员变量 mcontext 和 arr1 中。
这个适配器类还包含一个 ViewHolder 内部类,它用于缓存 RecyclerView 中的列表项视图。实现 RecyclerView.Adapter<MemoAdapter.ViewHolder> 接口,它的泛型参数指定了 ViewHolder 类型。在 onBindViewHolder() 方法中,数据绑定到 ViewHolder 的视图中。
如果您有任何关于 MemoAdapter 类的问题,请在回复中提供更多的细节和完整的代码。我会尽力帮助您解决问题。
阅读全文
相关推荐
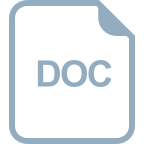
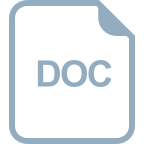
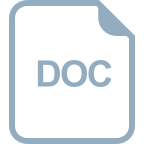














