编写一个java程序,实现二叉树排序
时间: 2023-09-14 21:06:12 浏览: 73
### 回答1:
很高兴为您提供帮助!请参考以下代码:
public class BinaryTreeSort {
// 根结点
private Node root; // 构建二叉树的结构
public class Node {
int value;
Node left;
Node right; public Node(int value) {
this.value = value;
left = null;
right = null;
}
} // 插入新结点
public void insert(int value) {
root = insertRec(root, value);
} // 插入新结点,递归实现
private Node insertRec(Node root, int value) {
if (root == null) {
root = new Node(value);
return root;
}
if (value < root.value)
root.left = insertRec(root.left, value);
else if (value > root.value)
root.right = insertRec(root.right, value);
return root;
} // 中序遍历,实现排序
public void inOrder() {
inOrderRec(root);
} // 中序遍历,递归实现
private void inOrderRec(Node root) {
if (root != null) {
inOrderRec(root.left);
System.out.println(root.value);
inOrderRec(root.right);
}
}
}
### 回答2:
二叉树排序是一种常见的排序算法,可以通过构建一颗二叉搜索树来实现。以下是一个使用Java编写的二叉树排序程序的示例:
```java
// 定义二叉树节点类
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
left = null;
right = null;
}
}
// 定义二叉搜索树类
class BinarySearchTree {
private TreeNode root;
BinarySearchTree() {
root = null;
}
// 插入一个节点
private TreeNode insert(TreeNode root, int val) {
if (root == null) {
root = new TreeNode(val);
return root;
} else if (val < root.val) {
root.left = insert(root.left, val);
} else if (val > root.val) {
root.right = insert(root.right, val);
}
return root;
}
// 中序遍历二叉树,输出排序结果
private void inOrderTraversal(TreeNode root) {
if (root != null) {
inOrderTraversal(root.left);
System.out.print(root.val + " ");
inOrderTraversal(root.right);
}
}
// 对外提供排序方法
void sort(int[] arr) {
for (int val : arr) {
root = insert(root, val);
}
inOrderTraversal(root);
}
}
// 测试程序
public class BinaryTreeSort {
public static void main(String[] args) {
BinarySearchTree bst = new BinarySearchTree();
int[] arr = {7, 3, 9, 2, 4, 8, 10};
bst.sort(arr);
}
}
```
以上程序中,我们定义了一个`BinarySearchTree`类来表示二叉搜索树,通过调用`sort`方法可以对传入的整数数组进行二叉树排序,并通过中序遍历算法输出排序结果。在`BinarySearchTree`类中,我们使用`insert`方法来实现节点的插入操作,使用`inOrderTraversal`方法来进行中序遍历。最后,我们在`main`方法中创建了一个`BinarySearchTree`对象,并通过`sort`方法对整数数组进行排序并输出。
### 回答3:
以下是一个用Java编写的实现二叉树排序的程序:
```java
// 定义二叉树节点
class TreeNode {
int val;
TreeNode left;
TreeNode right;
public TreeNode(int val) {
this.val = val;
}
}
// 实现二叉树排序
class BinaryTreeSort {
// 向二叉树中插入一个节点
public TreeNode insert(TreeNode root, int val) {
if (root == null) {
return new TreeNode(val);
}
if (val < root.val) {
root.left = insert(root.left, val);
} else if (val > root.val) {
root.right = insert(root.right, val);
}
return root;
}
// 中序遍历二叉树,得到升序排序结果
public void inorderTraversal(TreeNode root) {
if (root == null) {
return;
}
inorderTraversal(root.left);
System.out.print(root.val + " ");
inorderTraversal(root.right);
}
}
// 主程序
class Main {
public static void main(String[] args) {
BinaryTreeSort treeSort = new BinaryTreeSort();
int[] arr = {5, 2, 8, 1, 3};
TreeNode root = null;
for (int num : arr) {
root = treeSort.insert(root, num);
}
System.out.println("排序结果:");
treeSort.inorderTraversal(root);
}
}
```
这个程序通过定义一个`TreeNode`类来表示二叉树的节点,然后使用`BinaryTreeSort`类实现二叉树的插入和中序遍历,最后在`Main`类中创建一个二叉树并进行排序。运行程序后,将输出升序排序的结果:
```
排序结果:
1 2 3 5 8
```
此程序利用二叉树的性质,每次插入新的节点时,比较节点值与当前节点的大小关系,然后递归地插入到左子树或右子树中。通过中序遍历可以得到升序排序的结果。
相关推荐
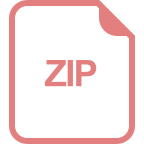














