python 多并发按月拉取elasticsearch数据
时间: 2023-08-12 07:19:24 浏览: 45
要按月拉取Elasticsearch数据,可以根据时间范围构造查询语句,并使用Python的多线程或多进程模块实现多并发。
以下是一个简单的多线程实现的示例代码:
```python
from elasticsearch import Elasticsearch
import threading
import datetime
# Elasticsearch连接信息
es = Elasticsearch(hosts=[{'host': 'localhost', 'port': 9200}])
# 需要拉取数据的月份列表
months = ['2022-01', '2022-02', '2022-03']
# 拉取数据的查询语句模板
query_template = {
"query": {
"bool": {
"must": [
{ "range": { "@timestamp": { "gte": "%s", "lt": "%s" } } }
]
}
}
}
# 每个线程要拉取的数据量
chunk_size = 1000
# 拉取数据的函数
def fetch_data(start, end):
query = query_template.copy()
query['query']['bool']['must'][0]['range']['@timestamp']['gte'] = start
query['query']['bool']['must'][0]['range']['@timestamp']['lt'] = end
res = es.search(index="my-index", body=query, size=chunk_size)
# 处理拉取到的数据
for hit in res['hits']['hits']:
do_something_with_hit(hit)
# 创建多个线程并启动
threads = []
for month in months:
start = datetime.datetime.strptime(month, '%Y-%m')
end = (start + datetime.timedelta(days=31)).strftime('%Y-%m-%d')
t = threading.Thread(target=fetch_data, args=(month, end))
threads.append(t)
t.start()
# 等待所有线程结束
for t in threads:
t.join()
```
在这个示例中,我们首先定义了要拉取的月份列表和每个线程要拉取的数据量。然后,我们根据时间范围构造了查询语句模板,并使用`threading`模块创建了多个线程,并分配给它们不同的时间范围进行数据拉取。最后,我们等待所有线程结束。
需要注意的是,由于每个月的天数不同,因此在构造时间范围时需要根据实际情况进行调整。此外,还要特别注意线程安全问题,以避免出现数据竞争等问题。
相关推荐
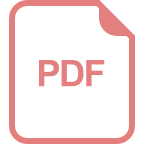














