Android studio设计备忘录,要求实现增删改查功能的代码
时间: 2024-01-22 16:20:12 浏览: 28
以下是一个简单的备忘录实现增删改查功能的代码,使用的是SQLite数据库:
```
public class MemoDatabaseHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "memo.db";
private static final int DATABASE_VERSION = 1;
public MemoDatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
String CREATE_MEMO_TABLE = "CREATE TABLE " + MemoContract.MemoEntry.TABLE_NAME + "("
+ MemoContract.MemoEntry._ID + " INTEGER PRIMARY KEY,"
+ MemoContract.MemoEntry.COLUMN_TITLE + " TEXT,"
+ MemoContract.MemoEntry.COLUMN_CONTENT + " TEXT,"
+ MemoContract.MemoEntry.COLUMN_TIMESTAMP + " DATETIME DEFAULT CURRENT_TIMESTAMP"
+ ")";
db.execSQL(CREATE_MEMO_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + MemoContract.MemoEntry.TABLE_NAME);
onCreate(db);
}
public long insertMemo(String title, String content) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(MemoContract.MemoEntry.COLUMN_TITLE, title);
values.put(MemoContract.MemoEntry.COLUMN_CONTENT, content);
long id = db.insert(MemoContract.MemoEntry.TABLE_NAME, null, values);
db.close();
return id;
}
public List<Memo> getAllMemos() {
List<Memo> memos = new ArrayList<>();
String selectQuery = "SELECT * FROM " + MemoContract.MemoEntry.TABLE_NAME + " ORDER BY " +
MemoContract.MemoEntry.COLUMN_TIMESTAMP + " DESC";
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.rawQuery(selectQuery, null);
if (cursor.moveToFirst()) {
do {
Memo memo = new Memo();
memo.setId(cursor.getInt(cursor.getColumnIndex(MemoContract.MemoEntry._ID)));
memo.setTitle(cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_TITLE)));
memo.setContent(cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_CONTENT)));
memo.setTimestamp(cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_TIMESTAMP)));
memos.add(memo);
} while (cursor.moveToNext());
}
db.close();
return memos;
}
public Memo getMemoById(long id) {
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = db.query(MemoContract.MemoEntry.TABLE_NAME,
new String[]{MemoContract.MemoEntry._ID, MemoContract.MemoEntry.COLUMN_TITLE,
MemoContract.MemoEntry.COLUMN_CONTENT, MemoContract.MemoEntry.COLUMN_TIMESTAMP},
MemoContract.MemoEntry._ID + "=?",
new String[]{String.valueOf(id)}, null, null, null, null);
if (cursor != null)
cursor.moveToFirst();
Memo memo = new Memo(
cursor.getInt(cursor.getColumnIndex(MemoContract.MemoEntry._ID)),
cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_TITLE)),
cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_CONTENT)),
cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_TIMESTAMP))
);
db.close();
return memo;
}
public int updateMemo(Memo memo) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(MemoContract.MemoEntry.COLUMN_TITLE, memo.getTitle());
values.put(MemoContract.MemoEntry.COLUMN_CONTENT, memo.getContent());
return db.update(MemoContract.MemoEntry.TABLE_NAME, values, MemoContract.MemoEntry._ID + " = ?",
new String[]{String.valueOf(memo.getId())});
}
public void deleteMemo(long id) {
SQLiteDatabase db = this.getWritableDatabase();
db.delete(MemoContract.MemoEntry.TABLE_NAME, MemoContract.MemoEntry._ID + " = ?",
new String[]{String.valueOf(id)});
db.close();
}
}
```
Memo.java:
```
public class Memo {
private int id;
private String title;
private String content;
private String timestamp;
public Memo() {
}
public Memo(int id, String title, String content, String timestamp) {
this.id = id;
this.title = title;
this.content = content;
this.timestamp = timestamp;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public String getTimestamp() {
return timestamp;
}
public void setTimestamp(String timestamp) {
this.timestamp = timestamp;
}
}
```
MemoContract.java:
```
public final class MemoContract {
private MemoContract() {}
public static class MemoEntry implements BaseColumns {
public static final String TABLE_NAME = "memo";
public static final String COLUMN_TITLE = "title";
public static final String COLUMN_CONTENT = "content";
public static final String COLUMN_TIMESTAMP = "timestamp";
}
}
```
然后在你的Activity或Fragment中,你可以如下使用:
```
public class MemoActivity extends AppCompatActivity {
private MemoDatabaseHelper dbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_memo);
dbHelper = new MemoDatabaseHelper(this);
// 插入备忘录
long memoId = dbHelper.insertMemo("Title", "Content");
// 获取所有备忘录
List<Memo> memos = dbHelper.getAllMemos();
// 获取单个备忘录
Memo memo = dbHelper.getMemoById(memoId);
// 更新备忘录
memo.setTitle("New Title");
dbHelper.updateMemo(memo);
// 删除备忘录
dbHelper.deleteMemo(memoId);
}
}
```
当然,你还需要在你的布局文件中添加相应的UI元素,例如EditText、ListView等等,以显示备忘录的内容。
相关推荐
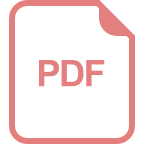
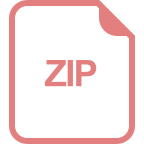














