LinuxC语言open
时间: 2023-06-13 19:06:38 浏览: 48
在Linux系统中,open()函数是用来打开文件的,其原型如下:
```c
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
```
其中,pathname是要打开的文件路径,flags是打开文件时的选项,包括:
- O_RDONLY:只读打开。
- O_WRONLY:只写打开。
- O_RDWR:读写打开。
- O_CREAT:如果文件不存在,就创建它。
- O_TRUNC:如果文件存在,就把文件长度截为0。
open()函数成功时返回一个文件描述符,失败则返回-1。文件描述符是一个非负整数,用来标识一个打开的文件。打开的文件需要在使用完后调用close()函数关闭。
例如,下面的代码打开一个名为test.txt的文件,并将其内容输出到终端:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#define BUF_SIZE 1024
int main(void)
{
int fd;
char buf[BUF_SIZE];
ssize_t num_read;
fd = open("test.txt", O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
while ((num_read = read(fd, buf, BUF_SIZE)) > 0) {
if (write(STDOUT_FILENO, buf, num_read) != num_read) {
perror("write");
exit(EXIT_FAILURE);
}
}
if (num_read == -1) {
perror("read");
exit(EXIT_FAILURE);
}
if (close(fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
return 0;
}
```
相关推荐
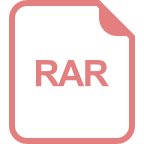
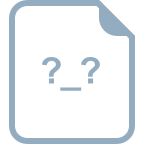














