opencv读取图像后使用resize和F.interpolate两种方法调整图像大小有什么区别
时间: 2024-06-11 11:11:07 浏览: 287
OpenCV中的resize函数和PyTorch中的F.interpolate函数都可以用来调整图像大小,但它们的使用方法和结果略有不同。
1. resize函数
resize函数可以直接调整图像的大小,并且可以选择不同的插值方法来处理图像的像素值。常用的插值方法有:INTER_NEAREST、INTER_LINEAR、INTER_CUBIC和INTER_LANCZOS4。
使用resize函数时,需要指定输出图像的大小和插值方法,例如:
```python
import cv2
img = cv2.imread('image.jpg')
resized_img = cv2.resize(img, (new_width, new_height), interpolation=cv2.INTER_LINEAR)
```
2. F.interpolate函数
F.interpolate函数是PyTorch中针对张量的插值函数,可以用于调整图像大小。与resize函数不同的是,F.interpolate函数需要将图像转换成PyTorch的张量,然后再进行插值操作。
使用F.interpolate函数时,需要指定输出张量的大小和插值方法,例如:
```python
import torch
import torch.nn.functional as F
img = cv2.imread('image.jpg')
tensor_img = torch.from_numpy(img).permute(2, 0, 1).unsqueeze(0).float()
resized_tensor_img = F.interpolate(tensor_img, size=(new_height, new_width), mode='bilinear', align_corners=True)
resized_img = resized_tensor_img.squeeze().permute(1, 2, 0).numpy().astype('uint8')
```
3. 区别
resize函数和F.interpolate函数的区别在于:
- resize函数可以直接操作图像,而F.interpolate需要将图像转换成张量后再操作。
- resize函数可以选择不同的插值方法,而F.interpolate只能选择双线性插值或最近邻插值。
- F.interpolate函数可以在GPU上进行加速,而resize函数只能在CPU上运行。
因此,如果只需要调整图像大小,可以使用resize函数;如果需要在PyTorch中进行深度学习,可以使用F.interpolate函数。
阅读全文
相关推荐
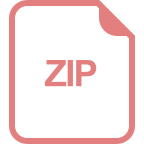
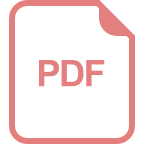
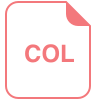
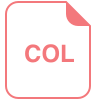
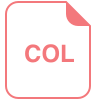
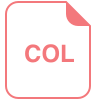
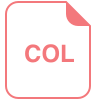

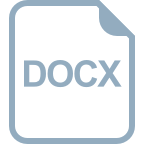
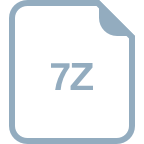
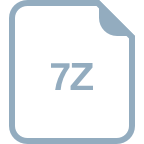
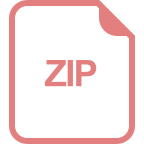
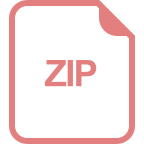