编程中途不要中断#include <iostream> #include <string> using namespace std; // 基类 Book class Book { public: // 构造函数 Book(string title, string author, int pages) : title(title), author(author), pages(pages) {} // 析构函数 ~Book() {} // 复制构造函数 Book(const Book& other) : title(other.title), author(other.author), pages(other.pages) {} // 友元函数,用于输出书籍信息 friend ostream& operator<<(ostream& os, const Book& b); // 静态函数,用于统计图书总数 static int getTotalBooks() { return totalBooks; } // 虚函数,用于多态输出 virtual void print() const; protected: string title; string author; int pages; static int totalBooks; // 静态数据成员,用于统计图书总数 }; int Book::totalBooks = 0; ostream& operator<<(ostream& os, const Book& b) { os << "书名:" << b.title << endl << "作者:" << b.author << endl << "页数:" << b.pages << endl; return os; } void Book::print() const { cout << *this << endl; } // 派生类 EBook,通过继承获得基类的所有成员 class EBook : public Book { public: // 构造函数 EBook(string title, string author, int pages, string format) : Book(title, author, pages), format(format) {} // 析构函数 ~EBook() {} // 复制构造函数 EBook(const EBook& other) : Book(other), format(other.format) {} // 重载输出运算符,用于输出电子书信息 friend ostream& operator<<(ostream& os, const EBook& eb); // 重写
时间: 2023-02-05 22:12:11 浏览: 123
好的,我会用中文回答你的问题。在编程中,中断通常指的是程序在运行过程中意外停止,这可能是由于程序出现了错误或者遇到了无法处理的异常情况。如果你想防止程序在中途中断,你可以尝试捕获异常,并在程序中处理这些异常,使程序能够继续运行下去。
阅读全文
相关推荐













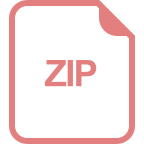
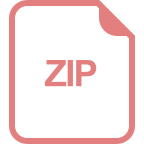
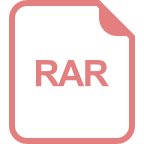