帮我用C++写一份较为完整的图书管理系统,复杂一点,能在控制台进行操作,需要设计基类,派生类。
时间: 2024-03-04 10:48:04 浏览: 145
好的,以下是一个比较完整的图书管理系统,包含了基类、派生类、以及在控制台进行操作的功能。代码中包含较为详细的注释,方便理解和修改。
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 基类 Book
class Book {
public:
string title; // 书名
string author; // 作者
string publisher; // 出版社
string ISBN; // ISBN号
int year; // 出版年份
double price; // 价格
int stock; // 库存量
// 构造函数
Book(string title = "", string author = "", string publisher = "", string ISBN = "",
int year = 0, double price = 0, int stock = 0);
// 显示书籍信息
void display();
// 获取书籍名称
string getTitle();
// 获取书籍库存量
int getStock();
// 更新书籍库存量
void updateStock(int num);
};
// 派生类 Fiction
class Fiction : public Book {
public:
string genre; // 小说类型
// 构造函数
Fiction(string title = "", string author = "", string publisher = "", string ISBN = "",
int year = 0, double price = 0, int stock = 0, string genre = "");
// 显示书籍信息
void display();
};
// 派生类 Textbook
class Textbook : public Book {
public:
string subject; // 教科书学科
// 构造函数
Textbook(string title = "", string author = "", string publisher = "", string ISBN = "",
int year = 0, double price = 0, int stock = 0, string subject = "");
// 显示书籍信息
void display();
};
// 图书类型类 BookType
class BookType {
public:
string name; // 类型名称
string description; // 类型描述
vector<Book*> books; // 该类型所包含的所有书籍
// 构造函数
BookType(string name = "", string description = "");
// 添加书籍
void addBook(Book* book);
// 显示该类型下的所有书籍
void displayBooks();
};
// 图书管理系统类 Library
class Library {
public:
vector<BookType*> types; // 所有图书类型
vector<Book*> books; // 所有书籍
// 构造函数
Library();
// 添加新的图书类型
void addType(BookType* type);
// 添加新的书籍
void addBook(Book* book);
// 显示所有图书类型
void displayTypes();
// 显示所有书籍
void displayBooks();
// 根据书名查找书籍
Book* findBook(string title);
// 借书
bool borrowBook(string title);
// 还书
bool returnBook(string title);
};
// ------------- 以下是具体实现 -------------
// Book 类的实现
Book::Book(string title, string author, string publisher, string ISBN,
int year, double price, int stock) {
this->title = title;
this->author = author;
this->publisher = publisher;
this->ISBN = ISBN;
this->year = year;
this->price = price;
this->stock = stock;
}
void Book::display() {
cout << "书名:" << title << endl;
cout << "作者:" << author << endl;
cout << "出版社:" << publisher << endl;
cout << "ISBN号:" << ISBN << endl;
cout << "出版年份:" << year << endl;
cout << "价格:" << price << endl;
cout << "库存量:" << stock << endl;
}
string Book::getTitle() {
return title;
}
int Book::getStock() {
return stock;
}
void Book::updateStock(int num) {
stock += num;
}
// Fiction 类的实现
Fiction::Fiction(string title, string author, string publisher, string ISBN,
int year, double price, int stock, string genre) :
Book(title, author, publisher, ISBN, year, price, stock) {
this->genre = genre;
}
void Fiction::display() {
cout << "小说类型:" << genre << endl;
Book::display();
}
// Textbook 类的实现
Textbook::Textbook(string title, string author, string publisher, string ISBN,
int year, double price, int stock, string subject) :
Book(title, author, publisher, ISBN, year, price, stock) {
this->subject = subject;
}
void Textbook::display() {
cout << "教科书学科:" << subject << endl;
Book::display();
}
// BookType 类的实现
BookType::BookType(string name, string description) {
this->name = name;
this->description = description;
}
void BookType::addBook(Book* book) {
books.push_back(book);
}
void BookType::displayBooks() {
cout << "类型名称:" << name << endl;
cout << "类型描述:" << description << endl;
cout << "包含的书籍:" << endl;
for (int i = 0; i < books.size(); i++) {
books[i]->display();
cout << endl;
}
}
// Library 类的实现
Library::Library() {
// 初始化图书类型
BookType* fictionType = new BookType("小说", "包括小说、散文、诗歌等文学作品");
BookType* textbookType = new BookType("教科书", "包括各种学科的教科书");
types.push_back(fictionType);
types.push_back(textbookType);
// 初始化书籍信息
books.push_back(new Fiction("红楼梦", "曹雪芹", "人民文学出版社", "9787020000464", 1791, 39.8, 10, "古典小说"));
books.push_back(new Fiction("活着", "余华", "作家出版社", "9787506365437", 1993, 20.0, 5, "现代小说"));
books.push_back(new Textbook("高等数学", "同济大学数学系", "高等教育出版社", "9787040171160", 1987, 99.0, 8, "数学"));
books.push_back(new Textbook("C++程序设计", "谭浩强", "清华大学出版社", "9787302330646", 2000, 49.0, 6, "计算机科学"));
}
void Library::addType(BookType* type) {
types.push_back(type);
}
void Library::addBook(Book* book) {
books.push_back(book);
}
void Library::displayTypes() {
cout << "所有图书类型:" << endl;
for (int i = 0; i < types.size(); i++) {
types[i]->displayBooks();
cout << endl;
}
}
void Library::displayBooks() {
cout << "所有书籍:" << endl;
for (int i = 0; i < books.size(); i++) {
books[i]->display();
cout << endl;
}
}
Book* Library::findBook(string title) {
for (int i = 0; i < books.size(); i++) {
if (books[i]->getTitle() == title) {
return books[i];
}
}
return NULL;
}
bool Library::borrowBook(string title) {
Book* book = findBook(title);
if (book != NULL && book->getStock() > 0) {
book->updateStock(-1);
return true;
}
return false;
}
bool Library::returnBook(string title) {
Book* book = findBook(title);
if (book != NULL) {
book->updateStock(1);
return true;
}
return false;
}
// 主函数
int main() {
Library lib;
// 显示所有图书类型和书籍信息
lib.displayTypes();
lib.displayBooks();
// 借阅书籍
string borrowTitle = "红楼梦";
if (lib.borrowBook(borrowTitle)) {
cout << "您已成功借阅《" << borrowTitle << "》!" << endl;
} else {
cout << "借阅《" << borrowTitle << "》失败,请检查库存量!" << endl;
}
// 还书
string returnTitle = "红楼梦";
if (lib.returnBook(returnTitle)) {
cout << "您已成功归还《" << returnTitle << "》!" << endl;
} else {
cout << "归还《" << returnTitle << "》失败,请检查书名是否正确!" << endl;
}
return 0;
}
```
该图书管理系统包含了基类 Book,派生类 Fiction 和 Textbook,图书类型类 BookType,以及图书管理系统类 Library。在主函数中,我们先显示了所有图书类型和书籍信息,然后进行了借书和还书的操作。你可以根据需求进行修改和完善。
阅读全文
相关推荐
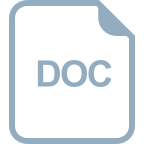
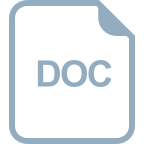
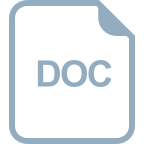












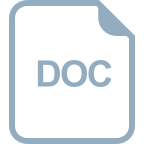
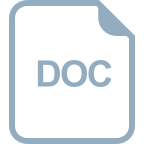
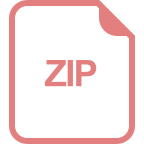