帮我用C++写一份较为完整的图书管理系统,能在控制台进行操作,需要基类,派生类,要能进行数据的保存和读取。
时间: 2024-03-04 14:48:13 浏览: 107
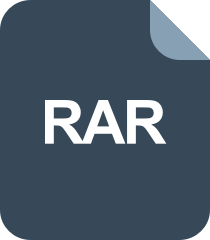
一个C++编写的图书管理系统

好的,这是一个比较复杂的项目,需要一步一步来完成。首先,我们需要设计类的继承关系。在本项目中,我们可以将图书馆中的书籍分为两类:普通书籍和期刊杂志。因此,我们可以设计一个基类 Book,然后派生出两个类,分别为 OrdinaryBook 和 Magazine。
接下来,我们需要考虑如何进行数据的保存和读取。这里,我建议使用文件来进行存储,可以将每个书籍的信息存储在一个单独的文件中,方便进行管理。在读取数据时,我们可以遍历文件夹,将每个文件中的信息读取出来,然后创建对应的对象,加入到图书馆中。
下面是一个简单的代码示例,仅供参考:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
class Book {
public:
Book(string name, string author, string publisher, int year) : m_name(name), m_author(author), m_publisher(publisher), m_year(year) {};
virtual void display() = 0;
virtual void saveToFile(string filename) = 0;
virtual void readFromFile(string filename) = 0;
protected:
string m_name;
string m_author;
string m_publisher;
int m_year;
};
class OrdinaryBook : public Book {
public:
OrdinaryBook(string name, string author, string publisher, int year, int pages) : Book(name, author, publisher, year), m_pages(pages) {};
virtual void display() {
cout << "书名:" << m_name << endl;
cout << "作者:" << m_author << endl;
cout << "出版社:" << m_publisher << endl;
cout << "出版年份:" << m_year << endl;
cout << "页数:" << m_pages << endl;
}
virtual void saveToFile(string filename) {
ofstream fout(filename);
fout << "ordinary_book" << endl;
fout << m_name << endl;
fout << m_author << endl;
fout << m_publisher << endl;
fout << m_year << endl;
fout << m_pages << endl;
fout.close();
}
virtual void readFromFile(string filename) {
ifstream fin(filename);
string type;
getline(fin, type);
getline(fin, m_name);
getline(fin, m_author);
getline(fin, m_publisher);
fin >> m_year;
fin >> m_pages;
fin.close();
}
private:
int m_pages;
};
class Magazine : public Book {
public:
Magazine(string name, string author, string publisher, int year, int issue) : Book(name, author, publisher, year), m_issue(issue) {};
virtual void display() {
cout << "杂志名:" << m_name << endl;
cout << "主编:" << m_author << endl;
cout << "出版社:" << m_publisher << endl;
cout << "出版年份:" << m_year << endl;
cout << "期号:" << m_issue << endl;
}
virtual void saveToFile(string filename) {
ofstream fout(filename);
fout << "magazine" << endl;
fout << m_name << endl;
fout << m_author << endl;
fout << m_publisher << endl;
fout << m_year << endl;
fout << m_issue << endl;
fout.close();
}
virtual void readFromFile(string filename) {
ifstream fin(filename);
string type;
getline(fin, type);
getline(fin, m_name);
getline(fin, m_author);
getline(fin, m_publisher);
fin >> m_year;
fin >> m_issue;
fin.close();
}
private:
int m_issue;
};
class Library {
public:
void addBook(Book* book) {
m_books.push_back(book);
}
void displayAllBooks() {
for (int i = 0; i < m_books.size(); i++) {
cout << "编号:" << i << endl;
m_books[i]->display();
cout << endl;
}
}
void saveAllBooks() {
for (int i = 0; i < m_books.size(); i++) {
string filename = "book_" + to_string(i) + ".txt";
m_books[i]->saveToFile(filename);
}
}
void readAllBooks() {
m_books.clear();
int i = 0;
while (true) {
string filename = "book_" + to_string(i) + ".txt";
ifstream fin(filename);
if (!fin.good()) {
break;
}
string type;
getline(fin, type);
if (type == "ordinary_book") {
string name, author, publisher;
int year, pages;
getline(fin, name);
getline(fin, author);
getline(fin, publisher);
fin >> year;
fin >> pages;
OrdinaryBook* book = new OrdinaryBook(name, author, publisher, year, pages);
m_books.push_back(book);
}
else if (type == "magazine") {
string name, author, publisher;
int year, issue;
getline(fin, name);
getline(fin, author);
getline(fin, publisher);
fin >> year;
fin >> issue;
Magazine* book = new Magazine(name, author, publisher, year, issue);
m_books.push_back(book);
}
fin.close();
i++;
}
}
private:
vector<Book*> m_books;
};
int main() {
Library library;
library.readAllBooks();
while (true) {
cout << "请输入操作编号:" << endl;
cout << "1. 添加书籍" << endl;
cout << "2. 显示所有书籍" << endl;
cout << "3. 保存所有书籍" << endl;
cout << "4. 退出" << endl;
int choice;
cin >> choice;
if (choice == 1) {
cout << "请输入书籍类型(1. 普通书籍,2. 期刊杂志):" << endl;
int type;
cin >> type;
cout << "请输入书名:" << endl;
string name;
cin >> name;
cout << "请输入作者:" << endl;
string author;
cin >> author;
cout << "请输入出版社:" << endl;
string publisher;
cin >> publisher;
cout << "请输入出版年份:" << endl;
int year;
cin >> year;
if (type == 1) {
cout << "请输入页数:" << endl;
int pages;
cin >> pages;
Book* book = new OrdinaryBook(name, author, publisher, year, pages);
library.addBook(book);
}
else if (type == 2) {
cout << "请输入期号:" << endl;
int issue;
cin >> issue;
Book* book = new Magazine(name, author, publisher, year, issue);
library.addBook(book);
}
}
else if (choice == 2) {
library.displayAllBooks();
}
else if (choice == 3) {
library.saveAllBooks();
}
else if (choice == 4) {
break;
}
}
return 0;
}
```
以上是一个简单的图书管理系统的实现,仅供参考。实际项目中,还需要考虑更多的细节问题,例如输入的合法性检查、异常处理等等。
阅读全文
相关推荐











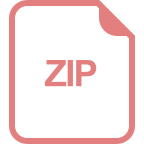
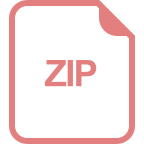
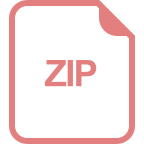
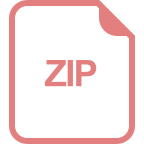
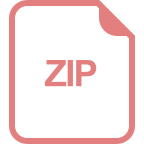