帮我用C++写一份较为完整的公司雇佣管理系统,复杂一点,代码需要详细的注释,能在控制台进行操作,需要设计基类,派生类。
时间: 2024-03-04 11:48:06 浏览: 75
很高兴能够帮您解决这个问题。
首先,公司雇佣管理系统需要设计基类和派生类,可以先设计一个基类Employee,包括姓名、性别、年龄、工资等基本信息,然后派生出Manager和Staff类分别代表经理和员工,添加特有的属性和方法。
下面是C++代码,注释较为详细:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Employee {
public:
Employee(string name, string gender, int age, int salary) {
this->name = name;
this->gender = gender;
this->age = age;
this->salary = salary;
}
// 基类的构造函数,初始化基本信息
virtual void showInfo() {
cout << "姓名:" << this->name << endl;
cout << "性别:" << this->gender << endl;
cout << "年龄:" << this->age << endl;
cout << "工资:" << this->salary << endl;
}
// 基类的成员函数,显示员工的基本信息
protected:
string name;
string gender;
int age;
int salary;
// 定义基础属性
};
class Manager : public Employee {
public:
Manager(string name, string gender, int age, int salary, string department) : Employee(name, gender, age, salary) {
this->department = department;
}
// 经理的构造函数,初始化经理的信息和基类的信息
void showInfo() override {
cout << "【经理】" << endl;
Employee::showInfo();
cout << "部门:" << this->department << endl;
}
// 经理的成员函数,显示经理信息
private:
string department;
// 定义经理特有的属性
};
class Staff : public Employee {
public:
Staff(string name, string gender, int age, int salary, string position) : Employee(name, gender, age, salary) {
this->position = position;
}
// 员工的构造函数,初始化员工的信息和基类的信息
void showInfo() override {
cout << "【员工】" << endl;
Employee::showInfo();
cout << "职位:" << this->position << endl;
}
// 员工的成员函数,显示员工信息
private:
string position;
// 定义员工特有的属性
};
int main() {
vector<Employee*> employees;
// 使用vector存储员工信息
Manager m1("张经理", "男", 35, 20000, "销售部");
Manager m2("王经理", "女", 40, 25000, "人事部");
Staff s1("李员工", "男", 25, 8000, "销售代表");
Staff s2("赵员工", "女", 28, 10000, "人事专员");
// 创建经理和员工对象,并初始化信息
employees.push_back(&m1);
employees.push_back(&m2);
employees.push_back(&s1);
employees.push_back(&s2);
// 将员工加入vector中
for (auto employee : employees) {
employee->showInfo();
cout << endl;
}
// 遍历vector,并调用showInfo函数显示员工信息
return 0;
}
```
上述代码中,通过vector存储员工信息,可以方便地进行添加、删除、修改等操作。Employee类作为基类,Manager和Staff类作为派生类,对基类进行了扩展,增加了特有的属性和方法。
在main函数中,先创建经理和员工对象,然后将它们加入vector中。最后遍历vector,并调用showInfo函数显示员工信息。
阅读全文
相关推荐
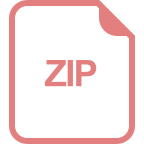
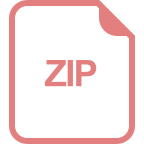
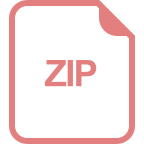















