帮我用C++写一份较为完整的图书管理系统,能在控制台进行操作,需要基类,派生类,要能进行数据的保存和读取。
时间: 2024-03-04 16:48:12 浏览: 72
好的,我可以为你提供一份基于 C++ 的图书管理系统代码。下面是代码实现的步骤:
1. 首先需要设计一个基类 Book,它包含以下数据成员:
- 书籍编号
- 书名
- 作者
- 出版社
- 出版日期
- 价格
同时,需要为这个基类设计以下成员函数:
- 构造函数,用于初始化书籍信息
- 修改书籍信息的函数
- 输出书籍信息的函数
- 获取书籍编号的函数
- 获取书籍价格的函数
代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
class Book {
protected:
int id;
string name;
string author;
string publisher;
string pubDate;
double price;
public:
Book(int id, string name, string author, string publisher, string pubDate, double price) {
this->id = id;
this->name = name;
this->author = author;
this->publisher = publisher;
this->pubDate = pubDate;
this->price = price;
}
virtual ~Book() {}
virtual void modify() {}
virtual void display() {}
int getId() { return id; }
double getPrice() { return price; }
};
```
2. 接下来需要设计一个派生类 BookManager,它包含以下数据成员:
- 书籍数组
- 书籍数量
同时,需要为这个派生类设计以下成员函数:
- 构造函数,用于初始化书籍数组
- 添加书籍信息的函数
- 修改书籍信息的函数
- 删除书籍信息的函数
- 按书籍编号查找书籍信息的函数
- 按价格排序书籍信息的函数
- 将书籍信息保存为文件的函数
- 从文件读取书籍信息的函数
代码如下:
```c++
#include <vector>
#include <algorithm>
#include <fstream>
class BookManager : public Book {
private:
vector<Book*> books;
int count;
public:
BookManager(int count) : Book(0, "", "", "", "", 0.0) {
this->count = count;
for (int i = 0; i < count; i++) {
books.push_back(nullptr);
}
}
~BookManager() {
for (auto book : books) {
if (book != nullptr) {
delete book;
}
}
}
void addBook() {
int id;
string name, author, publisher, pubDate;
double price;
cout << "请输入书籍编号:";
cin >> id;
cout << "请输入书名:";
cin >> name;
cout << "请输入作者:";
cin >> author;
cout << "请输入出版社:";
cin >> publisher;
cout << "请输入出版日期:";
cin >> pubDate;
cout << "请输入价格:";
cin >> price;
Book* book = new Book(id, name, author, publisher, pubDate, price);
for (int i = 0; i < count; i++) {
if (books[i] == nullptr) {
books[i] = book;
break;
}
}
cout << "添加成功!" << endl;
}
void modifyBook(int id) {
for (auto book : books) {
if (book != nullptr && book->getId() == id) {
book->modify();
cout << "修改成功!" << endl;
return;
}
}
cout << "未找到该书籍!" << endl;
}
void deleteBook(int id) {
for (auto book : books) {
if (book != nullptr && book->getId() == id) {
delete book;
book = nullptr;
cout << "删除成功!" << endl;
return;
}
}
cout << "未找到该书籍!" << endl;
}
void searchBook(int id) {
for (auto book : books) {
if (book != nullptr && book->getId() == id) {
book->display();
return;
}
}
cout << "未找到该书籍!" << endl;
}
void sortBook() {
sort(books.begin(), books.end(), [](Book* a, Book* b) {
if (a == nullptr || b == nullptr) {
return false;
}
return a->getPrice() < b->getPrice();
});
cout << "排序成功!" << endl;
}
void saveBook() {
ofstream file("books.txt");
for (auto book : books) {
if (book != nullptr) {
file << book->getId() << " " << book->name << " " << book->author << " " << book->publisher << " " << book->pubDate << " " << book->getPrice() << endl;
}
}
file.close();
cout << "保存成功!" << endl;
}
void loadBook() {
ifstream file("books.txt");
int id;
string name, author, publisher, pubDate;
double price;
while (file >> id >> name >> author >> publisher >> pubDate >> price) {
Book* book = new Book(id, name, author, publisher, pubDate, price);
for (int i = 0; i < count; i++) {
if (books[i] == nullptr) {
books[i] = book;
break;
}
}
}
file.close();
cout << "读取成功!" << endl;
}
};
```
3. 最后,我们需要在 main 函数中实现控制台的操作界面,包括添加书籍、修改书籍、删除书籍、查找书籍、排序书籍、保存书籍、读取书籍等操作。代码如下:
```c++
int main() {
BookManager bookManager(100);
int choice;
do {
cout << "1. 添加书籍" << endl;
cout << "2. 修改书籍" << endl;
cout << "3. 删除书籍" << endl;
cout << "4. 查找书籍" << endl;
cout << "5. 排序书籍" << endl;
cout << "6. 保存书籍" << endl;
cout << "7. 读取书籍" << endl;
cout << "0. 退出" << endl;
cout << "请选择操作:";
cin >> choice;
switch (choice) {
case 1:
bookManager.addBook();
break;
case 2:
int id;
cout << "请输入要修改的书籍编号:";
cin >> id;
bookManager.modifyBook(id);
break;
case 3:
cout << "请输入要删除的书籍编号:";
cin >> id;
bookManager.deleteBook(id);
break;
case 4:
cout << "请输入要查找的书籍编号:";
cin >> id;
bookManager.searchBook(id);
break;
case 5:
bookManager.sortBook();
break;
case 6:
bookManager.saveBook();
break;
case 7:
bookManager.loadBook();
break;
}
} while (choice != 0);
return 0;
}
```
以上就是基于 C++ 的图书管理系统的完整代码实现,希望对你有所帮助!
阅读全文
相关推荐
















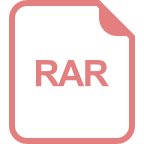
