用c++与easyx实现Nicholl-Lee-Nicholl线段裁剪算法
时间: 2024-03-20 15:42:11 浏览: 111
Nicholl-Lee-Nicholl(NLN)算法是一种线段裁剪算法,用于在计算机图形学中将线段裁剪到指定的窗口或视口中。它是一种快速而有效的算法,通常用于实时应用程序。
以下是使用C++和EasyX图形库实现NLN算法的代码:
```cpp
#include <graphics.h>
#include <iostream>
using namespace std;
// 定义 NLN 算法所需的几个数据结构
struct Point {
int x;
int y;
};
struct Edge {
Point p1;
Point p2;
float dx;
float dy;
};
struct Window {
int left;
int top;
int right;
int bottom;
};
// 定义 NLN 算法函数
void NLN(Edge edge, Window window) {
float t1 = 0, t2 = 1;
float dx = edge.dx;
float dy = edge.dy;
// 判断线段是否在窗口内
if (dx == 0) {
if ((edge.p1.x < window.left) || (edge.p1.x > window.right)) return;
} else {
float m = dy / dx;
float c = edge.p1.y - m * edge.p1.x;
float tleft = (window.left - c) / m;
float tright = (window.right - c) / m;
if (dx > 0) {
if (tleft > t1) t1 = tleft;
if (tright < t2) t2 = tright;
} else {
if (tleft < t2) t2 = tleft;
if (tright > t1) t1 = tright;
}
}
if (dy == 0) {
if ((edge.p1.y < window.top) || (edge.p1.y > window.bottom)) return;
} else {
float m = dx / dy;
float c = edge.p1.x - m * edge.p1.y;
float ttop = (window.top - c) / m;
float tbottom = (window.bottom - c) / m;
if (dy > 0) {
if (ttop > t1) t1 = ttop;
if (tbottom < t2) t2 = tbottom;
} else {
if (ttop < t2) t2 = ttop;
if (tbottom > t1) t1 = tbottom;
}
}
// 判断线段是否被裁剪
if (t1 <= t2) {
Point new_p1, new_p2;
new_p1.x = edge.p1.x + t1 * dx;
new_p1.y = edge.p1.y + t1 * dy;
new_p2.x = edge.p1.x + t2 * dx;
new_p2.y = edge.p1.y + t2 * dy;
// 绘制裁剪后的线段
line(new_p1.x, new_p1.y, new_p2.x, new_p2.y);
}
}
int main() {
// 创建图形窗口
initgraph(640, 480);
// 绘制窗口边框
setcolor(WHITE);
rectangle(100, 100, 500, 400);
// 定义窗口和线段数据
Window window = {100, 100, 500, 400};
Edge edge = {{50, 200}, {600, 200}, 0, 0};
// 计算线段裁剪后的结果并绘制
NLN(edge, window);
// 关闭图形窗口
getch();
closegraph();
return 0;
}
```
以上代码中,我们首先定义了三个数据结构:`Point` 代表点的坐标,`Edge` 代表线段的两个端点,以及 `Window` 代表窗口的大小。
然后,我们实现了 `NLN` 函数,该函数接受一个线段和一个窗口作为输入,并计算线段在窗口内的裁剪结果。该函数的实现基于 NLN 算法的基本原理,即通过计算线段与窗口之间的交点来裁剪线段。
最后,我们在 `main` 函数中使用 `NLN` 函数计算线段的裁剪结果,并绘制在图形窗口中。在绘制之前,我们还绘制了一个矩形边框来表示窗口的大小。
运行程序后,将看到裁剪后的线段仅在窗口内被绘制,而窗口外的部分被裁剪掉了。
阅读全文
相关推荐
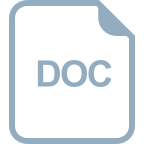
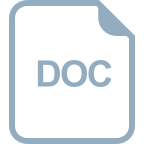
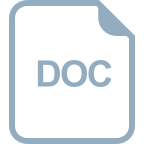

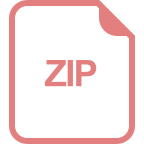
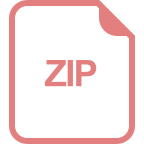

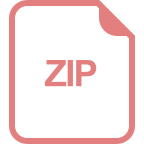
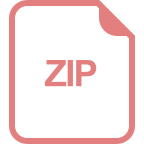
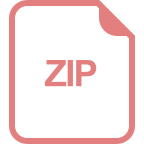
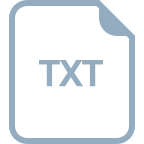
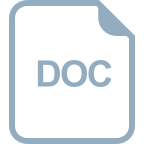
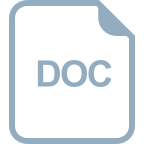


