根据上述思路用c++与easyx实现直线裁剪算法
时间: 2023-12-13 07:04:11 浏览: 41
好的,这里是一个简单的C++代码实现直线裁剪算法(Cohen-Sutherland算法)的示例,使用EasyX图形库进行绘图:
```cpp
#include <graphics.h>
#include <iostream>
using namespace std;
// 定义区域编码常量
const int INSIDE = 0; // 0000
const int LEFT = 1; // 0001
const int RIGHT = 2; // 0010
const int BOTTOM = 4; // 0100
const int TOP = 8; // 1000
// 定义窗口边界
const int XMIN = 100;
const int YMIN = 100;
const int XMAX = 500;
const int YMAX = 400;
// 定义直线端点
int x1, y1, x2, y2;
// 计算区域编码
int computeCode(int x, int y) {
int code = INSIDE;
if (x < XMIN) // 左
code |= LEFT;
else if (x > XMAX) // 右
code |= RIGHT;
if (y < YMIN) // 下
code |= BOTTOM;
else if (y > YMAX) // 上
code |= TOP;
return code;
}
// 裁剪直线
void clipLine(int& x1, int& y1, int& x2, int& y2) {
int code1 = computeCode(x1, y1);
int code2 = computeCode(x2, y2);
bool accept = false;
while (true) {
if (!(code1 | code2)) { // 全部在窗口内
accept = true;
break;
}
else if (code1 & code2) { // 全部在窗口外
break;
}
else { // 部分在窗口内
int code = code1 ? code1 : code2;
int x, y;
if (code & TOP) { // 与上边界相交
x = x1 + (x2 - x1) * (YMAX - y1) / (y2 - y1);
y = YMAX;
}
else if (code & BOTTOM) { // 与下边界相交
x = x1 + (x2 - x1) * (YMIN - y1) / (y2 - y1);
y = YMIN;
}
else if (code & RIGHT) { // 与右边界相交
y = y1 + (y2 - y1) * (XMAX - x1) / (x2 - x1);
x = XMAX;
}
else { // 与左边界相交
y = y1 + (y2 - y1) * (XMIN - x1) / (x2 - x1);
x = XMIN;
}
if (code == code1) {
x1 = x;
y1 = y;
code1 = computeCode(x1, y1);
}
else {
x2 = x;
y2 = y;
code2 = computeCode(x2, y2);
}
}
}
if (accept) {
line(x1, y1, x2, y2);
}
}
int main() {
initgraph(600, 500);
setbkcolor(WHITE);
cleardevice();
x1 = 50;
y1 = 50;
x2 = 550;
y2 = 450;
// 绘制窗口
rectangle(XMIN, YMIN, XMAX, YMAX);
// 绘制直线
line(x1, y1, x2, y2);
// 裁剪直线
clipLine(x1, y1, x2, y2);
getch();
closegraph();
return 0;
}
```
在这个示例中,我们使用Cohen-Sutherland算法对一条直线进行裁剪,并使用EasyX图形库绘制出裁剪前后的直线和窗口边界。
相关推荐
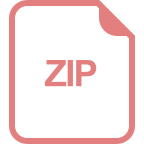














