利用c++和easyx库实现对一个不规则多边形Weler-Athenton多边形裁剪算法,请给出示例代码
时间: 2024-03-08 11:45:38 浏览: 91
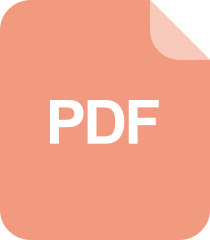
利用c++和easyx图形库做一个低配版扫雷游戏
好的,以下是利用C++和easyx库实现Weiler-Athenton多边形裁剪算法的示例代码:
```c++
#include <graphics.h>
#include <conio.h>
#include <vector>
// 定义点结构体
struct Point
{
int x;
int y;
Point() {}
Point(int x, int y) : x(x), y(y) {}
};
// 定义线段结构体
struct Segment
{
Point start;
Point end;
Segment() {}
Segment(Point start, Point end) : start(start), end(end) {}
};
// 定义多边形结构体
struct Polygon
{
std::vector<Point> vertices;
std::vector<Segment> edges;
Polygon() {}
Polygon(std::vector<Point> vertices) : vertices(vertices)
{
int n = vertices.size();
for (int i = 0; i < n; i++)
{
int j = (i + 1) % n;
edges.push_back(Segment(vertices[i], vertices[j]));
}
}
};
// 定义边界矩形
const int LEFT = 100;
const int TOP = 100;
const int RIGHT = 500;
const int BOTTOM = 400;
// 计算点p是否在边界矩形内
bool inside(Point p)
{
return p.x >= LEFT && p.x <= RIGHT && p.y >= TOP && p.y <= BOTTOM;
}
// 计算点p和点q的交点
Point intersection(Point p, Point q, int edge)
{
Point r;
int x1 = LEFT;
int y1 = TOP;
int x2 = RIGHT;
int y2 = TOP;
if (edge == 1)
{
x1 = RIGHT;
y1 = TOP;
x2 = RIGHT;
y2 = BOTTOM;
}
else if (edge == 2)
{
x1 = RIGHT;
y1 = BOTTOM;
x2 = LEFT;
y2 = BOTTOM;
}
else if (edge == 3)
{
x1 = LEFT;
y1 = BOTTOM;
x2 = LEFT;
y2 = TOP;
}
if (q.x == p.x) // 垂直线段
{
r.x = q.x;
r.y = y1 + (y2 - y1) * (q.x - x1) / (x2 - x1);
}
else // 非垂直线段
{
float k = (float)(q.y - p.y) / (q.x - p.x);
float b = q.y - k * q.x;
r.y = k * x1 + b;
r.x = (r.y - b) / k;
}
return r;
}
// Weiler-Athenton多边形裁剪算法
Polygon clipPolygon(Polygon subject, Polygon clip)
{
// 计算多边形交点
std::vector<Point> intersections;
for (int i = 0; i < subject.edges.size(); i++)
{
Segment s = subject.edges[i];
for (int j = 0; j < clip.edges.size(); j++)
{
Segment c = clip.edges[j];
Point p = intersection(s.start, s.end, j);
if (inside(p))
{
intersections.push_back(p);
}
}
}
// 构造交点多边形
Polygon intersectionPolygon(intersections);
// 构造裁剪后的多边形
Polygon output;
for (int i = 0; i < clip.vertices.size(); i++)
{
Point c = clip.vertices[i];
int k = intersectionPolygon.vertices.size();
for (int j = 0; j < k; j++)
{
Point p1 = intersectionPolygon.vertices[j];
Point p2 = intersectionPolygon.vertices[(j + 1) % k];
Segment s(p1, p2);
if (s.start == c) // Case 1
{
if (output.vertices.empty() || output.vertices.back() != c)
{
output.vertices.push_back(c);
}
output.vertices.push_back(s.end);
}
else if (s.end == c) // Case 2
{
if (output.vertices.empty() || output.vertices.back() != c)
{
output.vertices.push_back(c);
}
output.vertices.push_back(s.start);
}
else if (inside(s.start)) // Case 3
{
if (output.vertices.empty() || output.vertices.back() != s.start)
{
output.vertices.push_back(s.start);
}
}
else if (inside(s.end)) // Case 4
{
if (output.vertices.empty() || output.vertices.back() != s.end)
{
output.vertices.push_back(s.end);
}
}
else // Case 5
{
// do nothing
}
}
}
return output;
}
int main()
{
initgraph(640, 480);
// 定义不规则多边形
std::vector<Point> subjectVertices = {
{200, 200},
{250, 150},
{300, 200},
{300, 300},
{200, 300}
};
Polygon subject(subjectVertices);
// 定义裁剪窗口
std::vector<Point> clipVertices = {
{LEFT, TOP},
{RIGHT, TOP},
{RIGHT, BOTTOM},
{LEFT, BOTTOM}
};
Polygon clip(clipVertices);
// 裁剪多边形
Polygon output = clipPolygon(subject, clip);
// 绘制不规则多边形
setcolor(BLUE);
for (int i = 0; i < subject.vertices.size(); i++)
{
int j = (i + 1) % subject.vertices.size();
line(subject.vertices[i].x, subject.vertices[i].y, subject.vertices[j].x, subject.vertices[j].y);
}
// 绘制裁剪窗口
setcolor(GREEN);
for (int i = 0; i < clip.vertices.size(); i++)
{
int j = (i + 1) % clip.vertices.size();
line(clip.vertices[i].x, clip.vertices[i].y, clip.vertices[j].x, clip.vertices[j].y);
}
// 绘制裁剪后的多边形
setcolor(RED);
for (int i = 0; i < output.vertices.size(); i++)
{
int j = (i + 1) % output.vertices.size();
line(output.vertices[i].x, output.vertices[i].y, output.vertices[j].x, output.vertices[j].y);
}
getch();
closegraph();
return 0;
}
```
这段代码实现了对一个不规则多边形的Weiler-Athenton多边形裁剪算法,并使用了easyx库进行图形绘制。具体实现过程在代码注释中有详细解释。
阅读全文
相关推荐
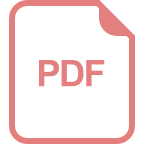
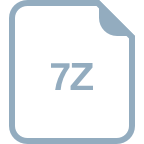















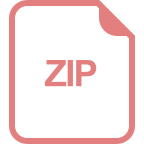