C++语言EasyX库VS2022边相关扫描算法代码
时间: 2023-08-16 20:03:39 浏览: 108
以下是使用EasyX库和VS2022编写的边相关扫描算法代码示例:
```c++
#include <graphics.h>
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Edge
{
int yMin; // 边的下端点y坐标
int yMax; // 边的上端点y坐标
float x; // 边的当前扫描线x坐标
float k; // 边的斜率
};
bool cmp(const Edge& a, const Edge& b)
{
return a.yMin < b.yMin;
}
void drawPolygon(vector<int>& x, vector<int>& y)
{
setbkcolor(WHITE);
cleardevice();
setcolor(BLACK);
fillpoly(x.size(), &x[0], &y[0]);
}
void scanLine(vector<int>& x, vector<int>& y)
{
vector<Edge> edgeTable;
int n = x.size();
for (int i = 0; i < n; i++)
{
Edge e;
if (y[i] < y[(i + 1) % n])
{
e.yMin = y[i];
e.yMax = y[(i + 1) % n];
e.x = x[i];
e.k = (float)(x[(i + 1) % n] - x[i]) / (float)(y[(i + 1) % n] - y[i]);
}
else
{
e.yMin = y[(i + 1) % n];
e.yMax = y[i];
e.x = x[(i + 1) % n];
e.k = (float)(x[i] - x[(i + 1) % n]) / (float)(y[i] - y[(i + 1) % n]);
}
edgeTable.push_back(e);
}
sort(edgeTable.begin(), edgeTable.end(), cmp);
vector<int> activeList;
int y = edgeTable[0].yMin;
while (y <= edgeTable[edgeTable.size() - 1].yMax)
{
for (int i = 0; i < edgeTable.size(); i++)
{
if (edgeTable[i].yMin == y)
{
activeList.push_back(i);
}
else if (edgeTable[i].yMax == y)
{
for (int j = 0; j < activeList.size(); j++)
{
if (activeList[j] == i)
{
activeList.erase(activeList.begin() + j);
break;
}
}
}
}
sort(activeList.begin(), activeList.end(), [&](int a, int b) {return edgeTable[a].x < edgeTable[b].x; });
for (int i = 0; i < activeList.size(); i += 2)
{
int x1 = (int)(edgeTable[activeList[i]].x + 0.5);
int x2 = (int)(edgeTable[activeList[i + 1]].x + 0.5);
line(x1, y, x2, y);
}
for (int i = 0; i < activeList.size(); i++)
{
edgeTable[activeList[i]].x += edgeTable[activeList[i]].k;
}
y++;
Sleep(20);
}
}
int main()
{
initgraph(640, 480);
vector<int> x = { 150, 300, 250, 100 };
vector<int> y = { 150, 150, 300, 300 };
drawPolygon(x, y);
scanLine(x, y);
_getch();
closegraph();
return 0;
}
```
该代码实现了对多边形进行边相关扫描算法,并使用EasyX库在窗口中显示出扫描结果。其中,`drawPolygon`函数用于绘制多边形,`scanLine`函数用于对多边形进行扫描,并在窗口中显示扫描结果。具体实现细节可以查看代码注释。
阅读全文
相关推荐
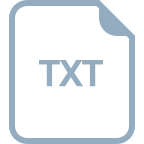

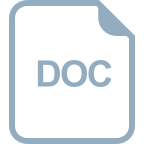







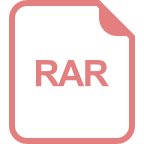
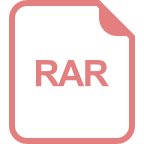
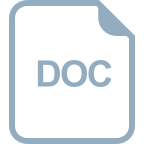
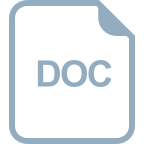
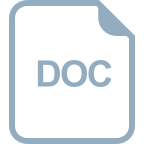