vue实现文件上传和下载
时间: 2024-06-10 13:10:50 浏览: 129
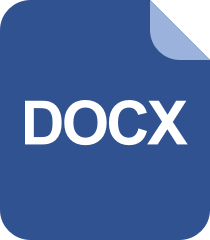
vue上传和下载前后台开发
文件上传和下载是前端开发中非常常见的功能,Vue提供了方便的方法来实现这些功能。
文件上传:
Vue中可以使用第三方库vue-file-upload来实现文件上传,具体步骤如下:
1. 安装vue-file-upload
```sh
npm install vue-file-upload --save
```
2. 在组件中引入并注册vue-file-upload
```js
import Vue from 'vue';
import VueFileUpload from 'vue-file-upload';
Vue.use(VueFileUpload);
```
3. 在模板中使用vue-file-upload来实现文件上传
```html
<template>
<div>
<vue-file-upload
url="/api/upload"
@success="onUploadSuccess"
@error="onUploadError"
@progress="onUploadProgress"
></vue-file-upload>
</div>
</template>
```
其中,url属性指定上传文件的地址,success、error和progress分别指定上传成功、上传失败和上传进度的回调函数。
文件下载:
Vue中可以使用原生的XMLHttpRequest对象或者第三方库axios来实现文件下载,具体步骤如下:
1. 使用XMLHttpRequest对象下载文件
```js
const xhr = new XMLHttpRequest();
xhr.open('GET', '/api/download', true);
xhr.responseType = 'blob';
xhr.onload = () => {
if (xhr.status === 200) {
const blob = new Blob([xhr.response], { type: 'application/octet-stream' });
const url = URL.createObjectURL(blob);
const link = document.createElement('a');
link.href = url;
link.download = 'file.txt';
link.click();
URL.revokeObjectURL(url);
}
};
xhr.send();
```
其中,responseType属性指定响应类型为blob,onload事件中将响应数据转为Blob对象,并创建一个超链接来下载文件。
2. 使用axios下载文件
```js
axios({
method: 'get',
url: '/api/download',
responseType: 'blob',
}).then((response) => {
const blob = new Blob([response.data], { type: 'application/octet-stream' });
const url = URL.createObjectURL(blob);
const link = document.createElement('a');
link.href = url;
link.download = 'file.txt';
link.click();
URL.revokeObjectURL(url);
});
```
其中,responseType属性指定响应类型为blob,然后将响应数据转为Blob对象,并创建一个超链接来下载文件。
阅读全文
相关推荐
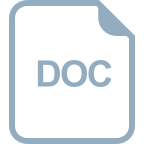
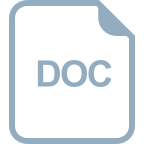
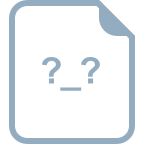
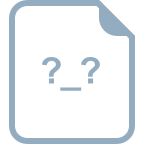
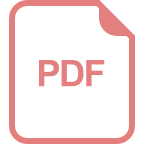
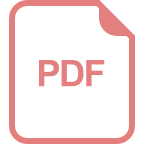
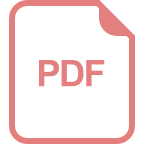


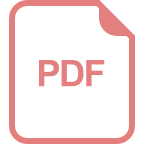
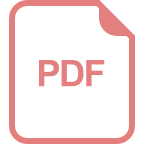
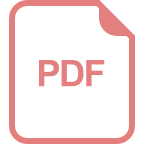





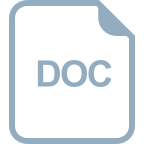