用、vue.js实现一个完整的购物车项目
时间: 2024-05-20 19:18:27 浏览: 132
好的,下面是一个简单的购物车项目的实现步骤,具体实现过程可以根据需求和技术栈的不同进行调整。
1. 创建一个Vue.js项目,可以使用Vue CLI工具进行创建,也可以手动搭建。
2. 在项目中安装并引入Vue Router和Vuex,分别用于路由管理和状态管理。
3. 创建一个商品列表组件,通过API调用获取商品数据并展示在页面上。
4. 在商品列表组件中添加一个“加入购物车”按钮,点击后将商品添加到购物车中。
5. 创建一个购物车组件,用于展示购物车中的商品列表和计算总价。
6. 在购物车组件中添加“删除”按钮,用于删除购物车中的商品。
7. 使用Vuex管理购物车状态,将购物车中的商品列表和总价存储在Vuex的状态中。
8. 使用Vue Router实现跳转到购物车页面。
9. 在购物车页面中展示购物车组件,并且从Vuex中获取购物车状态数据。
10. 完成购物车页面的样式设计,包括商品列表和总价的展示样式。
11. 最后,可以对项目进行优化,例如使用懒加载优化页面性能,添加loading效果等。
以上是一个简单的购物车项目的实现步骤,具体实现过程还需要根据实际需求进行调整。
相关问题
用vue.js语言实现一个完整的购物车项目
好的,下面是一个简单的购物车项目的实现,使用Vue.js框架和Bootstrap样式库。
首先,需要创建一个Vue实例,并定义一个数组来存储商品信息:
```html
<div id="app">
<div class="container">
<h1>购物车</h1>
<div class="row">
<div class="col-md-8">
<table class="table">
<thead>
<tr>
<th>商品</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in cartItems" :key="index">
<td>{{ item.name }}</td>
<td>{{ item.price }}</td>
<td>
<button class="btn btn-primary btn-sm" @click="decreaseQuantity(item)">-</button>
{{ item.quantity }}
<button class="btn btn-primary btn-sm" @click="increaseQuantity(item)">+</button>
</td>
<td>{{ item.price * item.quantity }}</td>
<td><button class="btn btn-danger btn-sm" @click="removeItem(item)">删除</button></td>
</tr>
</tbody>
</table>
</div>
<div class="col-md-4">
<h3>总计:{{ total }}</h3>
<button class="btn btn-success" @click="checkout">结算</button>
</div>
</div>
</div>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
cartItems: [
{ name: '商品1', price: 10, quantity: 1 },
{ name: '商品2', price: 20, quantity: 2 },
{ name: '商品3', price: 30, quantity: 3 }
]
},
computed: {
total: function() {
var sum = 0;
for (var i = 0; i < this.cartItems.length; i++) {
sum += this.cartItems[i].price * this.cartItems[i].quantity;
}
return sum;
}
},
methods: {
increaseQuantity: function(item) {
item.quantity++;
},
decreaseQuantity: function(item) {
item.quantity--;
if (item.quantity < 1) {
this.removeItem(item);
}
},
removeItem: function(item) {
var index = this.cartItems.indexOf(item);
if (index > -1) {
this.cartItems.splice(index, 1);
}
},
checkout: function() {
alert('结算成功,共计' + this.total + '元');
this.cartItems = [];
}
}
});
</script>
```
在Vue实例中,我们定义了一个`cartItems`数组,其中包含三个商品的信息,包括商品名称、单价和数量。在模板中,我们使用`v-for`指令来循环遍历数组,并将每个商品的信息显示在表格中。同时,我们还定义了`increaseQuantity`、`decreaseQuantity`和`removeItem`方法来增加、减少和删除商品数量,并计算总价。最后,我们定义了一个`checkout`方法来结算购物车并清空购物车数组。
这样就完成了一个简单的购物车项目的实现,你可以根据自己的需求来扩展和定制它。
用vue.js语言写一个购物车项目
好的,下面是一个简单的购物车项目示例,使用Vue.js语言编写:
1. 首先,安装Vue.js和Vue Router:
```
npm install vue
npm install vue-router
```
2. 创建一个Vue实例并使用Vue Router:
```
import Vue from 'vue'
import VueRouter from 'vue-router'
import App from './App.vue'
import Cart from './components/Cart.vue'
import Products from './components/Products.vue'
Vue.use(VueRouter)
const routes = [
{ path: '/cart', component: Cart },
{ path: '/products', component: Products }
]
const router = new VueRouter({
routes
})
new Vue({
render: h => h(App),
router
}).$mount('#app')
```
3. 创建一个App.vue组件,用于渲染整个应用程序:
```
<template>
<div id="app">
<router-link to="/cart">Cart</router-link>
<router-link to="/products">Products</router-link>
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'App'
}
</script>
<style>
#app {
font-family: Arial, Helvetica, sans-serif;
text-align: center;
}
</style>
```
4. 创建一个Cart.vue组件,用于显示购物车中的商品列表和总价:
```
<template>
<div>
<h2>Cart</h2>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.name }} - {{ item.price }} - {{ item.quantity }}
</li>
</ul>
<p>Total: ${{ total }}</p>
</div>
</template>
<script>
export default {
name: 'Cart',
data: () => ({
items: [
{ id: 1, name: 'Product 1', price: 10, quantity: 2 },
{ id: 2, name: 'Product 2', price: 20, quantity: 1 },
{ id: 3, name: 'Product 3', price: 30, quantity: 3 }
]
}),
computed: {
total() {
return this.items.reduce((acc, item) => acc + (item.price * item.quantity), 0)
}
}
}
</script>
```
5. 创建一个Products.vue组件,用于显示可供购买的商品列表和添加商品到购物车:
```
<template>
<div>
<h2>Products</h2>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.name }} - {{ item.price }} - <button @click="addItem(item)">Add to cart</button>
</li>
</ul>
</div>
</template>
<script>
export default {
name: 'Products',
data: () => ({
items: [
{ id: 1, name: 'Product 1', price: 10 },
{ id: 2, name: 'Product 2', price: 20 },
{ id: 3, name: 'Product 3', price: 30 }
]
}),
methods: {
addItem(item) {
this.$emit('add-to-cart', item)
alert('Added to cart: ' + item.name)
}
}
}
</script>
```
6. 在App.vue中添加一个购物车对象,以便从Products.vue中添加商品:
```
<template>
<div id="app">
<router-link to="/cart">Cart</router-link>
<router-link to="/products">Products</router-link>
<router-view @add-to-cart="addToCart"></router-view>
</div>
</template>
<script>
export default {
name: 'App',
data: () => ({
cart: []
}),
methods: {
addToCart(item) {
const existingItemIndex = this.cart.findIndex(cartItem => cartItem.id === item.id)
if (existingItemIndex !== -1) {
this.cart[existingItemIndex].quantity++
} else {
this.cart.push({ ...item, quantity: 1 })
}
}
}
}
</script>
```
7. 在Cart.vue中使用App.vue中的购物车对象来显示购物车中的商品:
```
<template>
<div>
<h2>Cart</h2>
<ul>
<li v-for="item in cart" :key="item.id">
{{ item.name }} - {{ item.price }} - {{ item.quantity }}
</li>
</ul>
<p>Total: ${{ total }}</p>
</div>
</template>
<script>
export default {
name: 'Cart',
computed: {
cart() {
return this.$root.cart
},
total() {
return this.$root.cart.reduce((acc, item) => acc + (item.price * item.quantity), 0)
}
}
}
</script>
```
这个简单的购物车项目示例演示了如何使用Vue.js语言编写一个具有路由和组件的应用程序,并在其中实现购物车功能。当然,你可以根据你的需求和喜好进行修改和扩展。
阅读全文
相关推荐
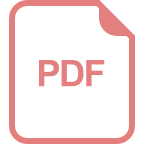
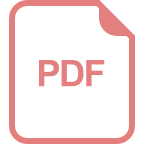
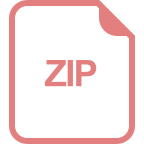













