1.读取名为“客户信息及违约表现”,格式为.xlsx的数据文件;提取特征变量和目标变量;将数据集划分为训练集和测试集;搭建决策树模型;训练模型;模型预测。 2.将上述决策树模型进行可视化呈现,展示图形结果。 3.对上述模型进行参数优化,返回最优参数和评分。代码
时间: 2024-03-25 11:39:16 浏览: 27
好的,以下是您需要的代码:
1. 读取Excel文件,提取特征变量和目标变量,划分数据集,搭建并训练决策树模型,模型预测:
```python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
# 读取Excel文件
df = pd.read_excel('客户信息及违约表现.xlsx')
# 提取特征变量和目标变量
X = df.iloc[:, 1:6]
y = df.iloc[:, 6]
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=0)
# 搭建决策树模型
clf = DecisionTreeClassifier()
# 训练模型
clf.fit(X_train, y_train)
# 模型预测
y_pred = clf.predict(X_test)
```
2. 将决策树模型可视化呈现:
```python
from sklearn.tree import export_graphviz
from graphviz import Source
# 导出决策树模型为Graphviz格式的数据
dot_data = export_graphviz(clf, out_file=None, feature_names=X.columns, class_names=['0', '1'], filled=True, rounded=True, special_characters=True)
# 将Graphviz格式的数据转换为图形结果
graph = Source(dot_data)
# 展示图形结果
graph
```
3. 对模型进行参数优化,返回最优参数和评分:
```python
from sklearn.model_selection import GridSearchCV
# 指定参数范围
param_grid = {'max_depth': [2, 4, 6, 8, 10], 'min_samples_split': [2, 4, 6, 8, 10]}
# 指定评分方法和交叉验证的折数
grid = GridSearchCV(clf, param_grid, scoring='accuracy', cv=5)
# 模型拟合和参数搜索
grid.fit(X_train, y_train)
# 返回最优参数和评分
print('最优参数:', grid.best_params_)
print('最优评分:', grid.best_score_)
```
希望对您有所帮助。
相关推荐
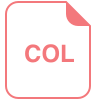
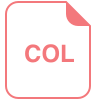
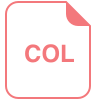
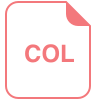
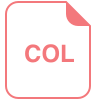










