opencv调用yolo
时间: 2023-11-06 07:04:33 浏览: 154
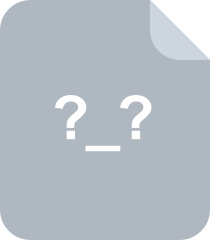
opencv-dnn模块调用YOLO模型进行目标检测
要使用OpenCV调用YOLO,需要先下载和安装YOLO的权重文件和配置文件,以及OpenCV的库文件。然后,可以使用以下代码加载模型并对图像进行目标检测:
```python
import cv2
# 加载YOLO模型
net = cv2.dnn.readNet("yolo.weights", "yolo.cfg")
# 读取图像
image = cv2.imread("test.jpg")
# 获取输入图像的尺寸
(H, W) = image.shape[:2]
# 构建输入图像的blob
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416), swapRB=True, crop=False)
# 设置模型的输入层
net.setInput(blob)
# 进行目标检测
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
outputs = net.forward(output_layers)
# 遍历输出层并获得检测结果
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * W)
center_y = int(detection[1] * H)
width = int(detection[2] * W)
height = int(detection[3] * H)
x = int(center_x - width / 2)
y = int(center_y - height / 2)
cv2.rectangle(image, (x, y), (x + width, y + height), (0, 255, 0), 2)
# 显示检测结果
cv2.imshow("Image", image)
cv2.waitKey(0)
```
需要注意的是,YOLO模型的输入图像尺寸必须为416x416,而且需要将图像归一化到0-1之间。此外,还需要根据YOLO的输出格式来解析检测结果。
阅读全文
相关推荐
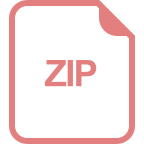















