逐行解释:typedef struct { int data[init_SIZE]; int top; } Stack;
时间: 2024-05-31 22:08:33 浏览: 22
1. typedef - 这个关键字表示定义一个新的数据类型名称。
2. struct - 这个关键字表示定义一个结构体类型。
3. int data[init_SIZE]; - 这个语句定义了一个整型数组 data,它有一个初始大小 init_SIZE。
4. int top; - 这个语句定义了一个整型变量 top,用于表示栈顶的位置。
5. } Stack; - 这个语句定义了一个名为 Stack 的结构体类型,包含一个整型数组 data 和一个整型变量 top。
相关问题
typedef struct { int data[init_SIZE]; int top; } Stack;
This code defines a structure named "Stack" which contains an integer array named "data" and an integer variable named "top". The "data" array is used to store the elements of the stack, and the "top" variable keeps track of the index of the top element in the stack. The initial size of the stack is defined by the constant "init_SIZE".
补全代码#include<stdio.h> #include<stdlib.h> typedef struct Stack{ int *data; int size, top; }Stack; Stack *init_stack(int n) { Stack *s = (Stack *)malloc(sizeof(Stack)); s->data = (int *)malloc(sizeof(int)); s->top = -1; s->size = n; return s; } void clear(Stack *s) { free(s->data); free(s); } int main() { return 0; }
这是一个基本的栈结构的定义和初始化函数以及清空函数的代码,但是缺少了栈的一些操作函数,比如 push()、pop()、peek() 等。可以参考下面的代码进行补充:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Stack{
int *data;
int size, top;
}Stack;
Stack *init_stack(int n) {
Stack *s = (Stack *)malloc(sizeof(Stack));
s->data = (int *)malloc(sizeof(int) * n);
s->top = -1;
s->size = n;
return s;
}
void clear(Stack *s) {
free(s->data);
free(s);
}
int push(Stack *s, int x) {
if (s->top == s->size - 1) {
return 0; // 栈满了
}
s->top++;
s->data[s->top] = x;
return 1;
}
int pop(Stack *s) {
if (s->top == -1) {
return 0; // 栈空了
}
s->top--;
return 1;
}
int peek(Stack *s) {
if (s->top == -1) {
return -1; // 栈空了
}
return s->data[s->top];
}
int main() {
Stack *s = init_stack(10);
push(s, 1);
push(s, 2);
push(s, 3);
printf("%d\n", peek(s)); // 输出3
pop(s);
printf("%d\n", peek(s)); // 输出2
clear(s);
return 0;
}
```
相关推荐
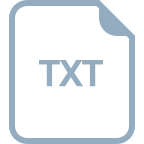
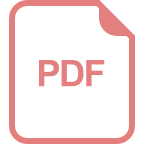
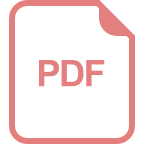












