我想通过complatefuture进行本地目录多线程打包zip,并将zip文件就返回前端怎么做
时间: 2024-04-03 10:34:29 浏览: 63
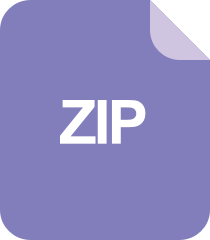
微前端集合,构建后的数据直接使用.zip
您可以使用Java的ZipOutputStream类将本地目录打包成zip文件,然后使用CompletableFuture异步处理文件打包过程。在CompletableFuture处理完毕后,您可以将打包好的zip文件返回给前端。
以下是一个简单的示例代码:
```java
public CompletableFuture<File> zipDirectory(String sourcePath, String zipPath) {
return CompletableFuture.supplyAsync(() -> {
try {
File sourceDir = new File(sourcePath);
File zipFile = new File(zipPath);
ZipOutputStream zipOut = new ZipOutputStream(new FileOutputStream(zipFile));
// Recursively add files to zip
addFilesToZip(zipOut, sourceDir, sourceDir.getName());
zipOut.close();
return zipFile;
} catch (IOException e) {
throw new RuntimeException("Failed to zip directory", e);
}
});
}
private void addFilesToZip(ZipOutputStream zipOut, File file, String parentName) throws IOException {
String name = parentName + "/" + file.getName();
if (file.isDirectory()) {
File[] files = file.listFiles();
for (File subFile : files) {
addFilesToZip(zipOut, subFile, name);
}
} else {
byte[] buffer = new byte[1024];
FileInputStream fis = new FileInputStream(file);
zipOut.putNextEntry(new ZipEntry(name));
int length;
while ((length = fis.read(buffer)) > 0) {
zipOut.write(buffer, 0, length);
}
fis.close();
}
}
```
您可以在您的控制器中调用这个方法来处理zip文件的打包:
```java
@RequestMapping("/zip")
public CompletableFuture<ResponseEntity<byte[]>> zipDirectory() {
String sourcePath = "/path/to/source/directory";
String zipPath = "/path/to/zip/file.zip";
return zipDirectory(sourcePath, zipPath)
.thenApply(file -> {
try {
byte[] bytes = Files.readAllBytes(file.toPath());
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDisposition(ContentDisposition.builder("attachment").filename(file.getName()).build());
return ResponseEntity.ok().headers(headers).body(bytes);
} catch (IOException e) {
throw new RuntimeException("Failed to read zip file", e);
}
});
}
```
这个控制器方法将返回一个`CompletableFuture<ResponseEntity<byte[]>>`对象,该对象在异步处理zip文件打包完成后,在响应中返回zip文件字节数组。在前端页面中,您可以将此字节数组转换为zip文件并进行下载。
阅读全文
相关推荐
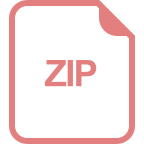
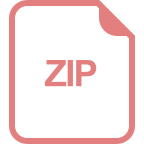
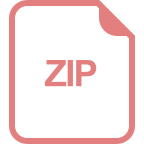
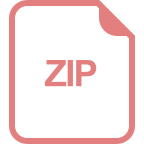
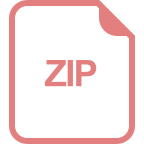
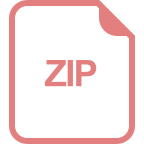
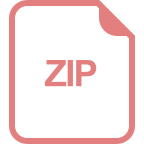
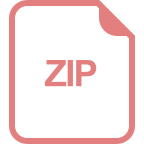
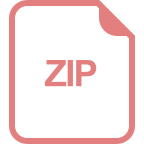
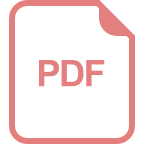