用JAVA语言写一个程序完成下面内容,并写好注释: 在几何上,有一种立体图形叫直棱柱(right prism),直棱柱的上下底面可以是圆形,三角形,四边形,五边形等等。 请创建一个抽象类RightPrism(构造方法为Shape类和double型高),以及抽象方法(体积公式calcVolume,侧面积公式calcLateralArea ),并发挥你的面向对象的思想,实现圆柱体、三棱柱、正方体、长方体。要求以 RightPrism xxx = new CircularCylinder ()这种多态的方式调用
时间: 2023-06-03 09:05:04 浏览: 69
:```
// 定义抽象类 RightPrism
public abstract class RightPrism extends Shape {
private double height;
public RightPrism(String name, double height) {
super(name);
this.height = height;
}
// 定义抽象方法,子类必须实现
public abstract double calcVolume();
public abstract double calcLateralArea();
public double getHeight() {
return height;
}
}
// 圆柱体类,继承 RightPrism
public class CircularCylinder extends RightPrism {
private double radius;
public CircularCylinder(String name, double height, double radius) {
super(name, height);
this.radius = radius;
}
// 实现抽象方法
public double calcVolume() {
return Math.PI * radius * radius * getHeight();
}
public double calcLateralArea() {
return 2 * Math.PI * radius * getHeight();
}
public double getRadius() {
return radius;
}
}
// 三棱柱类,继承 RightPrism
public class TriangularPrism extends RightPrism {
private double base;
private double side;
public TriangularPrism(String name, double height, double base, double side) {
super(name, height);
this.base = base;
this.side = side;
}
// 实现抽象方法
public double calcVolume() {
return base * getHeight() / 2 * side;
}
public double calcLateralArea() {
return side * getHeight() + base * getHeight() +
Math.sqrt(Math.pow(base, 2) + Math.pow(side, 2)) * getHeight();
}
public double getBase() {
return base;
}
public double getSide() {
return side;
}
}
// 正方体类,继承 RightPrism
public class Cube extends RightPrism {
private double edge;
public Cube(String name, double height, double edge) {
super(name, height);
this.edge = edge;
}
// 实现抽象方法
public double calcVolume() {
return Math.pow(edge, 3);
}
public double calcLateralArea() {
return 4 * edge * getHeight();
}
public double getEdge() {
return edge;
}
}
// 长方体类,继承 RightPrism
public class RectangularPrism extends RightPrism {
private double length;
private double width;
public RectangularPrism(String name, double height, double length, double width) {
super(name, height);
this.length = length;
this.width = width;
}
// 实现抽象方法
public double calcVolume() {
return length * width * getHeight();
}
public double calcLateralArea() {
return 2 * (length + width) * getHeight();
}
public double getLength() {
return length;
}
public double getWidth() {
return width;
}
}
// 使用多态调用
RightPrism cylinder = new CircularCylinder("圆柱体", 5.0, 3.0);
RightPrism triangularPrism = new TriangularPrism("三棱柱", 4.0, 6.0, 5.0);
RightPrism cube = new Cube("正方体", 2.0, 4.0);
RightPrism rectangularPrism = new RectangularPrism("长方体", 8.0, 5.0, 6.0);
System.out.println(cylinder.getName() + " 的体积为:" + cylinder.calcVolume());
System.out.println(triangularPrism.getName() + " 的侧面积为:" + triangularPrism.calcLateralArea());
System.out.println(cube.getName() + " 的体积为:" + cube.calcVolume());
System.out.println(rectangularPrism.getName() + " 的体积为:" + rectangularPrism.calcVolume());
```
相关推荐
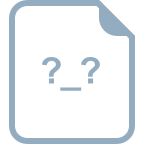
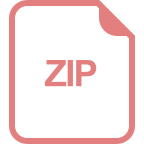
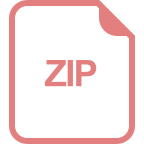














