用java写一个带有jframe中文操作页面的银行存取款业务,并附带注释
时间: 2023-11-27 09:53:24 浏览: 76
以下是一个简单的Java银行存取款业务的示例代码,使用JFrame构建中文操作页面,附带注释:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class BankApplication extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JLabel balanceLabel;
private JTextField amountField;
private JButton depositButton, withdrawButton;
private double balance = 0.0;
public BankApplication() {
// 设置窗口标题和大小
super("银行存取款业务");
setSize(300, 200);
// 创建界面元素
balanceLabel = new JLabel("当前余额: " + balance);
amountField = new JTextField(10);
depositButton = new JButton("存款");
withdrawButton = new JButton("取款");
// 将界面元素添加到窗口中
JPanel panel = new JPanel();
panel.add(balanceLabel);
panel.add(new JLabel("金额: "));
panel.add(amountField);
panel.add(depositButton);
panel.add(withdrawButton);
add(panel);
// 绑定事件处理程序
depositButton.addActionListener(this);
withdrawButton.addActionListener(this);
}
// 处理按钮点击事件
public void actionPerformed(ActionEvent e) {
String amountString = amountField.getText();
double amount = Double.parseDouble(amountString);
if (e.getSource() == depositButton) {
balance += amount;
} else if (e.getSource() == withdrawButton) {
balance -= amount;
}
balanceLabel.setText("当前余额: " + balance);
}
public static void main(String[] args) {
BankApplication frame = new BankApplication();
frame.setVisible(true);
}
}
```
代码中实现了一个简单的银行存取款业务,用户可以通过界面输入存款或取款金额,程序会根据用户的选择更新账户余额,并在界面上显示当前余额。在构建界面时,我们使用了JPanel来容纳界面元素,通过addActionListener()方法将事件处理程序绑定到按钮上,实现了简单的交互式操作。
阅读全文
相关推荐
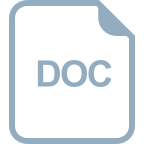
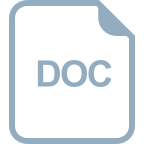
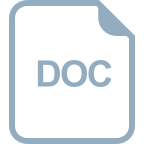



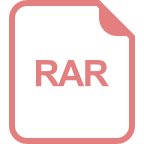
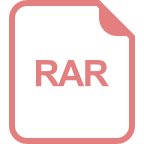
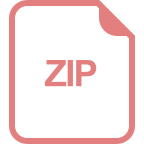
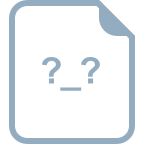
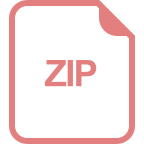
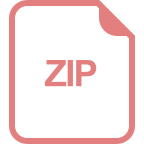



