请帮我写一段C++代码读取ini文件
时间: 2023-05-22 15:05:52 浏览: 107
当然可以帮您写一段C代码读取ini文件。首先,您需要知道ini文件的格式:它由[sections]和name=value的键值对组成。下面是一个简单的ini文件的例子:
```
; 注释
[section1]
key1 = value1
key2 = value2
[section2]
key3 = value3
key4 = value4
```
下面是读取ini文件的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LENGTH 256
#define MAX_SECTION_LENGTH 32
#define MAX_KEY_LENGTH 32
#define MAX_VALUE_LENGTH 128
typedef struct {
char section[MAX_SECTION_LENGTH];
char key[MAX_KEY_LENGTH];
char value[MAX_VALUE_LENGTH];
} IniEntry;
// 去除左右空格
char* trim(char *str) {
char *end;
while (isspace(*str)) str++;
if (*str == 0)
return str;
end = str + strlen(str) - 1;
while (end > str && isspace(*end)) end--;
*(end + 1) = 0;
return str;
}
// 读取ini文件并解析成条目数组
int read_ini_file(const char *filename, IniEntry *entries, int max_entries) {
FILE *file;
char buffer[MAX_LINE_LENGTH];
char *section = NULL;
int count = 0;
if ((file = fopen(filename, "r")) == NULL) {
printf("Failed to open the file: %s\n", filename);
return 0;
}
while (fgets(buffer, MAX_LINE_LENGTH, file) != NULL && count < max_entries) {
char *p = trim(buffer);
// 空行和注释行
if (!*p || *p == '#') continue;
// 节
if (*p == '[') {
char *q = strchr(p, ']');
if (q != NULL) {
*q = 0;
strncpy(entries[count].section, p + 1, MAX_SECTION_LENGTH);
entries[count].section[MAX_SECTION_LENGTH - 1] = 0;
section = entries[count].section;
}
}
// 键值对
else if (section != NULL) {
char *q = strchr(p, '=');
if (q != NULL) {
*q = 0;
strncpy(entries[count].key, p, MAX_KEY_LENGTH);
entries[count].key[MAX_KEY_LENGTH - 1] = 0;
strncpy(entries[count].value, q + 1, MAX_VALUE_LENGTH);
entries[count].value[MAX_VALUE_LENGTH - 1] = 0;
count++;
}
}
}
fclose(file);
return count;
}
int main(int argc, char *argv[]) {
IniEntry entries[256];
int count, i;
count = read_ini_file("test.ini", entries, 256);
for (i = 0; i < count; i++) {
printf("[%s]\n%s = %s\n\n", entries[i].section, entries[i].key, entries[i].value);
}
return 0;
}
```
您可以尝试将上述代码保存为ini.c,然后编译运行。
阅读全文
相关推荐
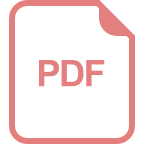
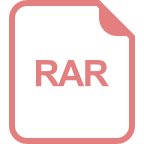
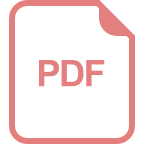













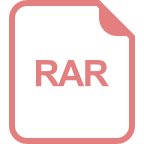
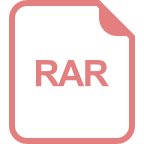
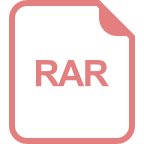