#include <ctype.h> int main() { char c; int alpha = 0, digit = 0, space = 0, other = 0; while ((c = getchar()) != '\n') { if (isalpha(c)) { alpha++; } else if (isdigit(c)) { digit++; } else if (isspace(c)) { space++; } else { other++; } } printf("%d %d %d %d\n", alpha, digit, space, other); return 0; }程序执行超时被强行终止,改进这段代码
时间: 2024-01-19 15:01:59 浏览: 37
这段代码的问题在于它每次读入一个字符后都需要进行一次判断,导致时间复杂度较高。改进的方法是每次读入一行字符,然后一次性对整个字符串进行统计。
以下是改进后的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_LEN 1024
int main() {
char line[MAX_LEN];
int alpha = 0, digit = 0, space = 0, other = 0;
while (fgets(line, MAX_LEN, stdin) != NULL) {
int len = strlen(line);
for (int i = 0; i < len; i++) {
char c = line[i];
if (isalpha(c)) {
alpha++;
} else if (isdigit(c)) {
digit++;
} else if (isspace(c)) {
space++;
} else {
other++;
}
}
}
printf("%d %d %d %d\n", alpha, digit, space, other);
return 0;
}
```
改进后的代码每次读入一行字符,然后使用一个 for 循环对整个字符串进行逐个字符的判断,从而避免了每次读入一个字符的低效率问题。
相关推荐
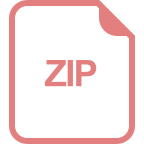
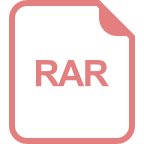














