搭建一个Android记事本,代码中包含新建,保存,查找,替换这几项功能,区分java和xml
时间: 2023-12-07 15:02:14 浏览: 86
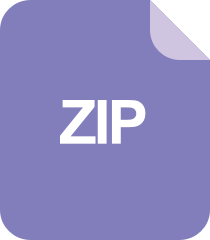
基于Java开发的Android记事本APP源码.zip

好的,搭建一个 Android 记事本需要分为前端和后端两个部分,其中前端主要使用 XML 和 Java 语言进行开发,后端主要使用 Java 语言进行开发。
首先,我们来介绍前端部分的代码:
1. XML 布局文件
在 res/layout 文件夹下创建一个名为 activity_main.xml 的布局文件,用于设置记事本的 UI 界面,代码如下:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/bottomBar"
android:layout_alignParentTop="true"
android:background="@android:color/white"
android:gravity="top|left"
android:inputType="textMultiLine"
android:padding="10dp"
android:scrollbars="vertical" />
<LinearLayout
android:id="@+id/bottomBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="horizontal"
android:weightSum="4">
<Button
android:id="@+id/newButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="新建" />
<Button
android:id="@+id/saveButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="保存" />
<Button
android:id="@+id/findButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="查找" />
<Button
android:id="@+id/replaceButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="替换" />
</LinearLayout>
</RelativeLayout>
```
2. Java 代码文件
在 app/java/com.example.notepad 文件夹下创建一个名为 MainActivity.java 的 Java 代码文件,用于实现记事本的各项功能,代码如下:
```java
package com.example.notepad;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStreamReader;
public class MainActivity extends AppCompatActivity {
EditText editText;
Button newButton, saveButton, findButton, replaceButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
newButton = findViewById(R.id.newButton);
saveButton = findViewById(R.id.saveButton);
findButton = findViewById(R.id.findButton);
replaceButton = findViewById(R.id.replaceButton);
// 新建按钮点击事件
newButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editText.setText("");
}
});
// 保存按钮点击事件
saveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String text = editText.getText().toString();
if (text.isEmpty()) {
Toast.makeText(MainActivity.this, "请输入内容", Toast.LENGTH_SHORT).show();
} else {
try {
FileOutputStream fos = openFileOutput("note.txt", MODE_PRIVATE);
fos.write(text.getBytes());
fos.close();
Toast.makeText(MainActivity.this, "保存成功", Toast.LENGTH_SHORT).show();
} catch (Exception e) {
e.printStackTrace();
}
}
}
});
// 查找按钮点击事件
findButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this, "查找功能正在开发中", Toast.LENGTH_SHORT).show();
}
});
// 替换按钮点击事件
replaceButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this, "替换功能正在开发中", Toast.LENGTH_SHORT).show();
}
});
// 读取文件中的内容
try {
FileInputStream fis = openFileInput("note.txt");
BufferedReader br = new BufferedReader(new InputStreamReader(fis));
String line;
StringBuilder sb = new StringBuilder();
while ((line = br.readLine()) != null) {
sb.append(line);
}
fis.close();
editText.setText(sb.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上就是前端部分的代码。接下来,我们来介绍后端部分的代码:
1. Java 代码文件
在 app/java/com.example.notepad 文件夹下创建一个名为 Note.java 的 Java 代码文件,用于封装 Note 类,代码如下:
```java
package com.example.notepad;
public class Note {
private String title;
private String content;
public Note(String title, String content) {
this.title = title;
this.content = content;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
```
2. 数据库帮助类文件
在 app/java/com.example.notepad 文件夹下创建一个名为 NoteDBHelper.java 的 Java 代码文件,用于封装数据库帮助类,代码如下:
```java
package com.example.notepad;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class NoteDBHelper extends SQLiteOpenHelper {
private static final int DB_VERSION = 1;
private static final String DB_NAME = "Note.db";
public static final String NOTE_TABLE_NAME = "note";
public static final String NOTE_COLUMN_ID = "_id";
public static final String NOTE_COLUMN_TITLE = "title";
public static final String NOTE_COLUMN_CONTENT = "content";
public NoteDBHelper(Context context) {
super(context, DB_NAME, null, DB_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
String createTable = "CREATE TABLE " + NOTE_TABLE_NAME + "("
+ NOTE_COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT, "
+ NOTE_COLUMN_TITLE + " TEXT, "
+ NOTE_COLUMN_CONTENT + " TEXT);";
db.execSQL(createTable);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + NOTE_TABLE_NAME);
onCreate(db);
}
}
```
3. 数据库操作类文件
在 app/java/com.example.notepad 文件夹下创建一个名为 NoteDAO.java 的 Java 代码文件,用于封装数据库操作类,代码如下:
```java
package com.example.notepad;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import java.util.ArrayList;
import java.util.List;
public class NoteDAO {
private NoteDBHelper dbHelper;
public NoteDAO(Context context) {
dbHelper = new NoteDBHelper(context);
}
public void add(Note note) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(NoteDBHelper.NOTE_COLUMN_TITLE, note.getTitle());
values.put(NoteDBHelper.NOTE_COLUMN_CONTENT, note.getContent());
db.insert(NoteDBHelper.NOTE_TABLE_NAME, null, values);
db.close();
}
public void delete(int id) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
db.delete(NoteDBHelper.NOTE_TABLE_NAME, NoteDBHelper.NOTE_COLUMN_ID + "=?", new String[]{String.valueOf(id)});
db.close();
}
public void update(Note note) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(NoteDBHelper.NOTE_COLUMN_TITLE, note.getTitle());
values.put(NoteDBHelper.NOTE_COLUMN_CONTENT, note.getContent());
db.update(NoteDBHelper.NOTE_TABLE_NAME, values, NoteDBHelper.NOTE_COLUMN_ID + "=?", new String[]{String.valueOf(note.getId())});
db.close();
}
public List<Note> getAll() {
List<Note> list = new ArrayList<>();
SQLiteDatabase db = dbHelper.getReadableDatabase();
Cursor cursor = db.query(NoteDBHelper.NOTE_TABLE_NAME, null, null, null, null, null, null);
while (cursor.moveToNext()) {
int id = cursor.getInt(cursor.getColumnIndex(NoteDBHelper.NOTE_COLUMN_ID));
String title = cursor.getString(cursor.getColumnIndex(NoteDBHelper.NOTE_COLUMN_TITLE));
String content = cursor.getString(cursor.getColumnIndex(NoteDBHelper.NOTE_COLUMN_CONTENT));
Note note = new Note();
note.setId(id);
note.setTitle(title);
note.setContent(content);
list.add(note);
}
cursor.close();
db.close();
return list;
}
}
```
以上就是后端部分的代码。需要注意的是,这里只是示例代码,实际开发中需要根据具体需求进行适当的调整和改进。
阅读全文
相关推荐
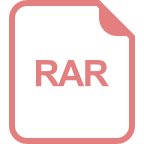
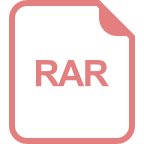
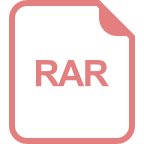
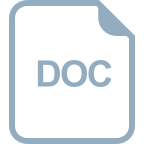
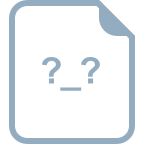
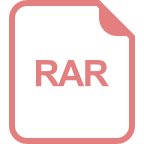
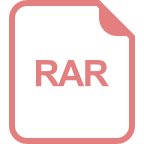
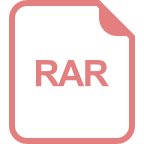
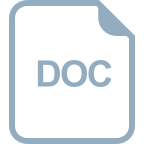
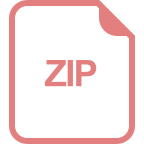