用visualstudio,opencv2实现RGB图片转化为三维float数组后通过数组显示RGB图片
时间: 2024-05-04 20:18:43 浏览: 9
以下是基于Visual Studio 2019和OpenCV 2.4.13的RGB图像转换为三维float数组并通过数组显示RGB图像的示例代码:
```c++
#include <iostream>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
using namespace std;
using namespace cv;
int main()
{
// 读取RGB图像
Mat image = imread("test.jpg", CV_LOAD_IMAGE_COLOR);
if (!image.data) {
cout << "Could not open or find the image" << endl;
return -1;
}
// 将RGB图像转换为三维float数组
int height = image.rows;
int width = image.cols;
int channels = image.channels();
float*** floatArray = new float**[height];
for (int i = 0; i < height; i++) {
floatArray[i] = new float*[width];
for (int j = 0; j < width; j++) {
floatArray[i][j] = new float[channels];
for (int k = 0; k < channels; k++) {
floatArray[i][j][k] = (float)image.at<Vec3b>(i, j)[k];
}
}
}
// 通过数组显示RGB图像
Mat result(height, width, CV_32FC3, Scalar(0, 0, 0));
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
for (int k = 0; k < channels; k++) {
result.at<Vec3f>(i, j)[k] = floatArray[i][j][k];
}
}
}
imshow("Result", result);
waitKey(0);
// 释放内存
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
delete[] floatArray[i][j];
}
delete[] floatArray[i];
}
delete[] floatArray;
return 0;
}
```
首先,我们使用OpenCV的`imread`函数读取RGB图像。接下来,我们将RGB图像转换为三维float数组。最后,我们使用OpenCV的`imshow`函数显示结果图像。请注意,我们使用了三层循环来遍历三维数组,并使用`at`函数访问图像像素。
请注意,此示例仅适用于像素值介于0和255之间的图像。如果您的图像像素值超出此范围,则需要进行归一化以将像素值缩放到0到1之间。
相关推荐
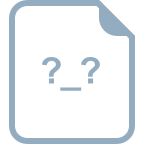
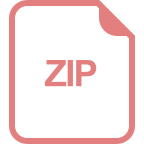
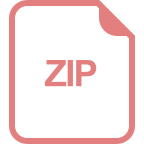
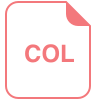
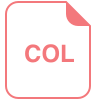
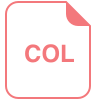
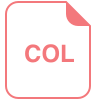










