Why the following programs will cause error? #include <iostream> using namespace std; template <typename T> T max(T x, T y) { return (x > y)? x : y; } int main() { cout << max(3, 7) << endl; cout << max(3.0, 7.0) << endl; cout << max(3, 7.0) << endl; return 0; }
时间: 2024-02-03 16:02:32 浏览: 36
The program will cause an error in the third `cout` statement because the types of the arguments passed to the `max()` function are different (`int` and `double`). The `max()` function is defined as a templated function that only takes arguments of the same type.
To fix it, you can either make sure that the arguments passed to the `max()` function are of the same type, or modify the `max()` function to take arguments of different types. Here's the corrected code using the latter approach, where the `max()` function takes arguments of different types using template specialization:
```
#include <iostream>
using namespace std;
template <typename T>
T max(T x, T y) {
return (x > y)? x : y;
}
template <>
double max(double x, int y) {
return (x > y)? x : y;
}
int main() {
cout << max(3, 7) << endl;
cout << max(3.0, 7.0) << endl;
cout << max(3, 7.0) << endl;
return 0;
}
```
In this corrected code, the `max()` function is specialized for the case where the first argument is a `double` and the second argument is an `int`. This allows the third `cout` statement to work correctly.
相关推荐
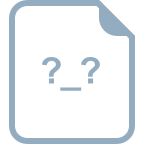














