Try to write an algorithm to Calculate the WPL of a Huffman Tree.
时间: 2024-01-13 14:03:10 浏览: 161
Here's an algorithm to calculate the weighted path length (WPL) of a Huffman tree:
1. Traverse the Huffman tree in a depth-first manner.
2. Assign a binary code of 0 to the left child and 1 to the right child of each node.
3. For each leaf node, multiply its depth (i.e., the length of the binary code) by its weight (i.e., the frequency of the corresponding character) to get its contribution to the WPL.
4. Sum up the contributions of all leaf nodes to get the total WPL.
Here's the pseudocode for the algorithm:
```
function calculate_wpl(huffman_tree):
wpl = 0
stack.push(huffman_tree.root)
while !stack.empty():
node = stack.pop()
if node.is_leaf():
wpl += node.depth * node.weight
else:
if node.left_child:
node.left_child.depth = node.depth + 1
node.left_child.code = node.code + '0'
stack.push(node.left_child)
if node.right_child:
node.right_child.depth = node.depth + 1
node.right_child.code = node.code + '1'
stack.push(node.right_child)
return wpl
```
Note that this algorithm assumes that the Huffman tree has already been constructed. If you need to construct the Huffman tree from scratch, you can use the Huffman coding algorithm.
阅读全文
相关推荐
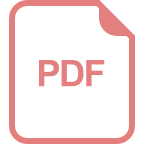
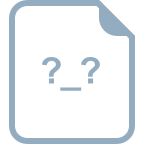
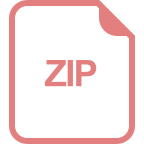
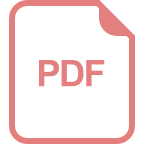
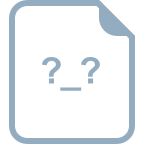
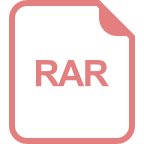
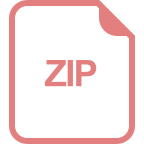
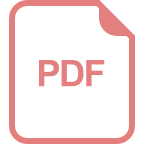
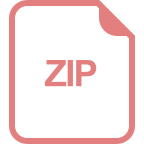
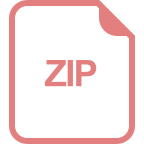
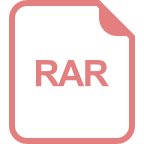
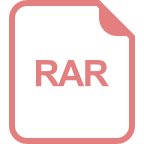
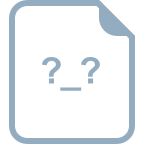
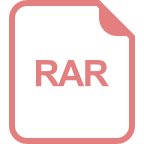