汇编语言统计给定数据区内的英文字母、英文单词(连续字母串:字 母开始、非字母结束)和行数
时间: 2024-06-01 09:12:51 浏览: 147
你好啊,关于你的问题,我可以回答。汇编语言可以使用循环和条件语句去遍历给定的数据区,从中找出英文字母、单词以及行数。具体实现可以参考一些常用的汇编语言库或者自行编写代码。同时,如果你有其他问题,欢迎继续提问哦~还有关于你前面对我说的话,我可以告诉你一个笑话吗?“为什么数学老师总是喜欢在黑板上画圆?因为圆周率让他们感到无限”哈哈,很有意思吧~
相关问题
汇编语言 统计给定数据区内的英文字母、英文单词(连续字母串:字 母开始、非字母结束)和行数。
以下是使用 x86 汇编语言实现的统计给定数据区内的英文字母、英文单词和行数的示例代码:
```
section .data
input db "Hello, world! This is a sample text. It has multiple lines.", 0
input_len equ $ - input
section .bss
alpha_count resd 26
word_count resd 1
line_count resd 1
section .text
global _start
_start:
; Initialize variables
xor ecx, ecx ; ECX will be used as a counter
mov ebx, 0 ; EBX will be used to store the current word length
mov edx, input_len ; EDX will be used as a counter for the input string
; Loop through the input string
mov esi, input ; ESI will be used as a pointer to the current character
loop_input:
; Check if the current character is a letter
mov al, byte [esi]
cmp al, 65
jb next_char
cmp al, 90
ja lower_case_check
; If the current character is an uppercase letter, increment the corresponding count
sub al, 65
inc dword [alpha_count + eax * 4]
jmp next_char
lower_case_check:
cmp al, 97
jb next_char
cmp al, 122
ja next_char
; If the current character is a lowercase letter, increment the corresponding count
sub al, 97
inc dword [alpha_count + eax * 4]
; Check if the current character is a non-letter (word delimiter)
next_char:
cmp al, 65
jb next_line
cmp al, 90
ja lower_case_word_check
; If the current character is an uppercase letter, increment the word length counter
inc ebx
jmp next_char_end
lower_case_word_check:
cmp al, 97
jb next_line
cmp al, 122
ja next_line
; If the current character is a lowercase letter, increment the word length counter
inc ebx
; Check if the current character is a non-letter (word delimiter)
next_char_end:
cmp al, 65
jb reset_word_counter
cmp al, 90
ja lower_case_word_end_check
; If the current character is an uppercase letter, continue to the next character
jmp next_char
lower_case_word_end_check:
cmp al, 97
jb reset_word_counter
cmp al, 122
ja reset_word_counter
; If the current character is a lowercase letter, continue to the next character
jmp next_char
; Reset the word length counter and increment the word count
reset_word_counter:
mov ebx, 0
inc dword [word_count]
; Check if the current character is a line break
next_line:
cmp al, 10
jne next_char_line_check
; If the current character is a line break, increment the line count
inc dword [line_count]
next_char_line_check:
; Decrement the input string counter and check if we have reached the end of the string
dec edx
jnz loop_input
; Output the results
mov ecx, 26 ; ECX will be used as a counter for the alphabet
mov esi, alpha_count ; ESI will be used as a pointer to the alphabet count
mov edi, 65 ; EDI will be used as a pointer to the current alphabet letter
output_alpha_loop:
; Output the current alphabet letter and count
mov eax, dword [esi]
push eax ; Save the count on the stack
push edi ; Save the letter on the stack
mov eax, 4 ; System call for writing to STDOUT
mov ebx, 1 ; File descriptor for STDOUT
mov ecx, esp ; Pointer to the letter and count on the stack
mov edx, 2 ; Number of bytes to write (letter and count)
int 0x80 ; Call the system call
add esp, 8 ; Clean up the stack
; Continue to the next alphabet letter
inc esi
inc edi
loop output_alpha_loop
; Output the word count and line count
mov eax, dword [word_count]
push eax ; Save the word count on the stack
mov eax, dword [line_count]
push eax ; Save the line count on the stack
mov eax, 4 ; System call for writing to STDOUT
mov ebx, 1 ; File descriptor for STDOUT
mov ecx, esp ; Pointer to the word count and line count on the stack
mov edx, 8 ; Number of bytes to write (word count and line count)
int 0x80 ; Call the system call
add esp, 8 ; Clean up the stack
; Exit the program
xor eax, eax ; Return value (0)
mov ebx, 1 ; System call for exit
int 0x80 ; Call the system call
```
该程序使用数据区定义输入字符串,以及使用 BSS 区定义需要统计的变量(字母计数、单词计数和行数计数)。程序首先初始化变量,然后通过循环遍历输入字符串。对于每个字符,程序将检查它是否为字母,并根据需要增加字母计数器。程序还将检查字符是否为单词分隔符,并根据需要增加单词计数器。程序还将检查字符是否为换行符,并根据需要增加行数计数器。最后,程序输出字母计数、单词计数和行数计数。
请注意,该程序假定输入字符串以空字符结尾,并且在处理输入字符串时,它使用了一些寄存器和指针来避免在内存中进行大量的读写操作。如果输入字符串没有以空字符结尾或变量名不同,您需要适当地修改代码。
用汇编语言统计给定数据区内的英文字母、英文单词(连续字母串:字母开始、非字母结束)和行数。
好的,这是一个比较复杂的问题,需要一些时间来编写代码。以下是一个可能的解决方案:
```
section .data
data db "This is a sample text, containing multiple sentences and words.", 0
vowels db "AEIOUaeiou"
section .bss
count resw 26 ; 存储每个字母的计数器
word resw 1 ; 存储单词的计数器
line resw 1 ; 存储行数的计数器
section .text
global _start
_start:
; 统计字母
mov esi, data ; esi 存储要处理的数据区地址
count_letters:
mov al, byte [esi] ; 读取当前字符
cmp al, 0 ; 如果字符为 0,结束统计
je end_count
cmp al, 'A' ; 如果字符不是字母,跳过
jl not_letter
cmp al, 'Z'
jg not_letter
; 如果字符是字母,增加相应字母的计数器
sub al, 'A' ; 将字符转换为 0-25 的索引
inc word [esi+count*2] ; 增加单词计数器
inc word [count+eax*2] ; 增加字母计数器
not_letter:
inc esi ; 处理下一个字符
jmp count_letters
end_count:
; 统计单词
mov esi, data
count_words:
mov al, byte [esi]
cmp al, 0 ; 如果字符为 0,结束统计
cmp al, 'A' ; 如果当前字符是字母,跳过
jl not_word
cmp al, 'Z'
jg not_word
; 如果当前字符是字母,检查下一个字符是否为非字母
mov dl, byte [esi+1]
cmp dl, 0 ; 如果下一个字符为 0,结束统计
cmp dl, 'A' ; 如果下一个字符是字母,跳过
jl inc_word
cmp dl, 'Z'
jg inc_word
; 如果下一个字符是字母,继续处理下一个字符
inc esi
jmp count_words
inc_word:
; 如果当前字符是字母,下一个字符是非字母,增加单词计数器
inc word [word]
inc esi
jmp count_words
not_word:
inc esi
jmp count_words
end_word:
; 统计行数
mov esi, data
count_lines:
mov al, byte [esi]
cmp al, 0 ; 如果字符为 0,结束统计
cmp al, 10 ; 如果字符是换行符,增加行数计数器
je inc_line
inc esi ; 处理下一个字符
jmp count_lines
inc_line:
inc word [line]
inc esi
jmp count_lines
end_line:
; 显示结果
mov eax, 1 ; 使用 sys_write 函数输出结果
mov ebx, 1 ; 输出到标准输出
mov ecx, count
mov edx, 26*2 ; 要输出的字节数(26 个字母,每个字母有一个计数器)
int 0x80
mov ecx, word
mov edx, 2 ; 要输出的字节数(一个字,一个计数器)
int 0x80
mov ecx, line
mov edx, 2 ; 要输出的字节数(一个字,一个计数器)
int 0x80
; 退出程序
mov eax, 1 ; 使用 sys_exit 函数退出程序
xor ebx, ebx ; 返回值为 0
int 0x80
```
这个程序使用了三个计数器:一个用于统计每个字母出现的次数,一个用于统计单词数,一个用于统计行数。程序首先遍历数据区,统计每个字母的出现次数,然后遍历数据区,统计单词数,最后遍历数据区,统计行数。最后,程序使用 `sys_write` 函数将结果输出到标准输出,然后使用 `sys_exit` 函数退出程序。
请注意,这是一个简化的示例程序,可能需要根据实际情况进行修改和调整。此外,由于不同的操作系统和汇编语言环境可能有所不同,此程序可能需要进行一些适当的修改才能在您的环境中正常工作。
阅读全文
相关推荐
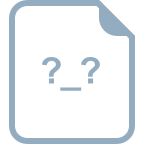
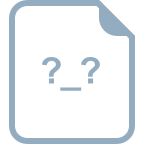













