Flutter 后台持续定位 高德
时间: 2023-06-13 13:06:29 浏览: 78
Flutter 可以通过使用高德地图 SDK 实现后台持续定位。以下是一些概述步骤:
1. 在 pubspec.yaml 文件中添加高德地图 SDK 的依赖。
2. 在 AndroidManifest.xml 文件中添加定位权限和服务声明。
3. 在 Info.plist 文件中添加定位权限请求。
4. 在 Flutter 中使用 MethodChannel 调用原生代码实现后台定位。
下面是一个示例代码:
```
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class LocationPage extends StatefulWidget {
@override
_LocationPageState createState() => _LocationPageState();
}
class _LocationPageState extends State<LocationPage> {
static const platform = const MethodChannel('com.example.location');
String location = '';
Future<void> _getLocation() async {
String result;
try {
result = await platform.invokeMethod('getLocation');
} on PlatformException catch (e) {
print(e);
}
setState(() {
location = result;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Location'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Location: $location'),
RaisedButton(
child: Text('Get Location'),
onPressed: _getLocation,
),
],
),
),
);
}
}
```
在原生代码中,可以使用高德地图 SDK 提供的 AMapLocationClient 类实现后台定位。以下是一个示例代码:
```
public class LocationPlugin implements MethodCallHandler {
private AMapLocationClient locationClient;
private AMapLocationListener locationListener;
public LocationPlugin(Context context) {
locationClient = new AMapLocationClient(context);
locationClient.setInterval(2000);
locationClient.setLocationOption(getDefaultOption());
locationListener = new AMapLocationListener() {
@Override
public void onLocationChanged(AMapLocation aMapLocation) {
if (aMapLocation != null) {
String location = String.format("%f,%f", aMapLocation.getLongitude(), aMapLocation.getLatitude());
sendLocationUpdate(location);
}
}
};
locationClient.setLocationListener(locationListener);
}
private AMapLocationClientOption getDefaultOption() {
AMapLocationClientOption option = new AMapLocationClientOption();
option.setLocationMode(AMapLocationClientOption.AMapLocationMode.Hight_Accuracy);
option.setInterval(2000);
option.setNeedAddress(false);
option.setWifiScan(true);
option.setKillProcess(false);
option.setSensorEnable(false);
option.setLocationCacheEnable(false);
return option;
}
private void sendLocationUpdate(String location) {
Intent intent = new Intent("com.example.location");
intent.putExtra("location", location);
LocalBroadcastManager.getInstance(context).sendBroadcast(intent);
}
@Override
public void onMethodCall(MethodCall call, Result result) {
switch (call.method) {
case "getLocation":
locationClient.startLocation();
break;
default:
result.notImplemented();
break;
}
}
}
```
在 AndroidManifest.xml 文件中,需要添加以下权限和服务声明:
```
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_BACKGROUND_LOCATION" />
<service android:name="com.example.location.LocationService" />
```
在 LocationService 类中,需要创建一个 LocationPlugin 对象,并在 onStartCommand 方法中启动后台定位:
```
public class LocationService extends Service {
private LocationPlugin locationPlugin;
@Override
public void onCreate() {
super.onCreate();
locationPlugin = new LocationPlugin(this);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
locationPlugin.startLocation();
return START_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
```
相关推荐
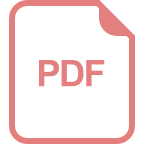
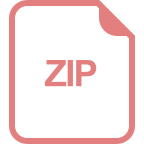














